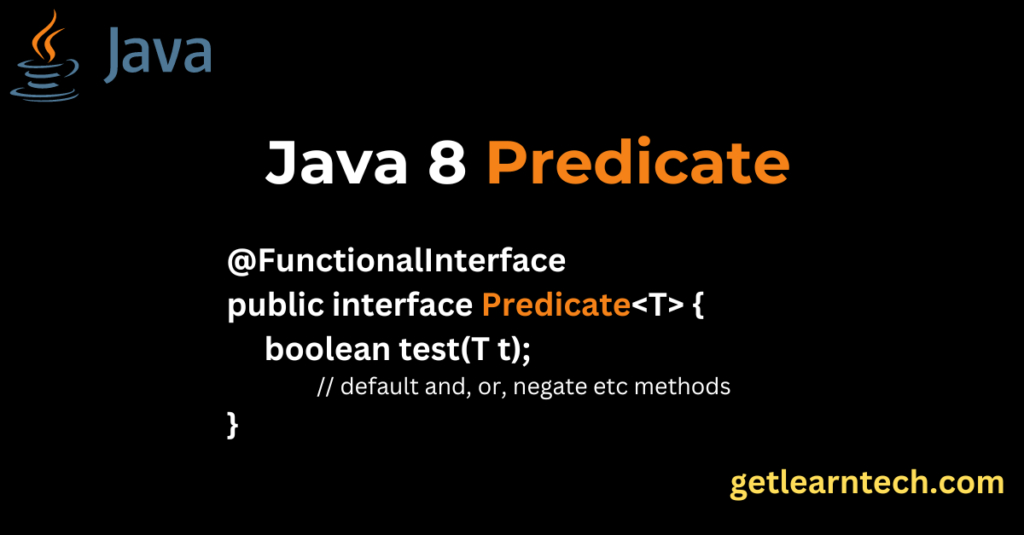
Table of Contents
Introduction
Java 8 introduced a new interface called Predicate, which is a functional interface that can be used to filter data based on certain conditions. Predicate is a powerful tool in Java that can help developers to simplify their code and make it more readable. In this post, we will discuss what is Java Predicate how to use it and its advantages.
Exploring Java 8 Predicate
Java 8 Predicate is a functional interface that is part of the java.util.function package. It takes one input parameter and returns a boolean value. It contains a single method called “test()” and it is used to evaluate the input parameter against a certain condition. Java 8 predicate with multiple arguments is not possible.
Java Predicate contains following methods
boolean test(T t)
This method is used to evaluate the predicate on a given input argument. It returns a boolean value indicating whether the predicate is true or false for the given input.
default Predicate<T> and(Predicate<? super T> other)
This method is used to create a new predicate that represents the logical AND of two predicates. It returns a new predicate that returns true only if both the original predicates are true for the given input.
default Predicate<T> negate()
This method is used to create a new predicate that represents the logical negation of the original predicate. It returns a new predicate that returns true when the original predicate returns false, and vice versa.
default Predicate<T> or(Predicate<? super T> other)
This method is used to create a new predicate that represents the logical OR of two predicates. It returns a new predicate that returns true if either of the original predicates are true for the given input.
static <T> Predicate<T> isEqual(Object targetRef)
This method is used to create a predicate that tests whether the input argument is equal to a given target reference. It returns a new predicate that returns true if the input argument is equal to the target reference, and false otherwise.
static <T> Predicate<T> not(Predicate<? super T> target)
In Java 11, Predicate
class introduced new method not()
. It returns a Predicate that is the negation of the supplied predicate. Internally, this is accomplished by returning the result of calling predicate.negate()
.
How to use Java 8 Predicate ?
To use Java Predicate, you first need to import the java.util.function package. Once you have imported the package, you can create a Predicate object by using the lambda expression.
Let us consider below simple Java 8 predicate example:
Predicate<Integer> isPositive = x -> x > 0;
In this example, we have created a Predicate object called “isPositive” that takes in an integer parameter and returns a boolean value based on whether the integer is positive or not.
You can then use the Predicate object to filter data. For example, if you have a list of integers, you can use the Predicate object to filter out the positive integers:
Predicate<Integer> isPositive = x -> x > 0;
System.out.println("Is positive "+ isPositive.test(-10)); // false
// In streams
List<Integer> numbers = Arrays.asList(-5, 0, 5, 10, -20);
List<Integer> positiveNumbers = numbers.stream()
.filter(isPositive)
.collect(Collectors.toList());
Java 8 predicate examples
Example 1 : Simple Predicate
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
public class SimplePredicateStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(5, 10, 15, 20, 25);
// Example: Predicate to check if a number is greater than 10
Predicate<Integer> isGreaterThanTen = num -> num > 10;
System.out.println("Is 12 greater then 10 "+ isGreaterThanTen.test(12));
// true
// Filter numbers greater than 10 using Predicate and Stream
numbers.stream()
.filter(isGreaterThanTen)
.forEach(System.out::println); // Output: 15, 20, 25
}
}
Example 2 : Predicate or()
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
public class PredicateWithOrStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(2, 4, 6, 8, 10, 12);
// Example: Predicate to check if a number is either less than 5 or greater than 10
Predicate<Integer> isLessThanFive = num -> num < 5;
Predicate<Integer> isGreaterThanTen = num -> num > 10;
Predicate<Integer> isLessThanFiveOrGreaterThanTen = isLessThanFive.or(isGreaterThanTen);
// Filter numbers less than 5 or greater than 10 using Predicate and Stream
numbers.stream()
.filter(isLessThanFiveOrGreaterThanTen)
.forEach(System.out::println); // Output: 2, 4, 12
}
}
Example 3 : Predicate and()
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
public class PredicateWithAndStreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(4, 6, 8, 10, 12);
// Example: Predicate to check if a number is both greater than 5 and even
Predicate<Integer> isGreaterThanFive = num -> num > 5;
Predicate<Integer> isEven = num -> num % 2 == 0;
Predicate<Integer> isGreaterThanFiveAndEven = isGreaterThanFive.and(isEven);
// Filter numbers greater than 5 and even using Predicate and Stream
numbers.stream()
.filter(isGreaterThanFiveAndEven)
.forEach(System.out::println); // Output: 6, 8, 10, 12
}
}
Example 4 : Predicate negate()
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
public class StreamingPredicateNegateExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Creating a predicate to check if a number is even
Predicate<Integer> isEven = num -> num % 2 == 0;
// Creating a predicate to negate the even check
Predicate<Integer> isOdd = isEven.negate();
List<Integer> oddNumbers = numbers.stream()
.filter(isOdd)
.collect(Collectors.toList());
// Output the filtered lists
System.out.println("Odd numbers: " + oddNumbers);
}
}
Example 5 : Predicate not() – this is introduced in Java 11
Predicate<Integer> isEven = i -> i % 2 == 0;
Predicate<Integer> isOdd = Predicate.not( isEven );
Example 6 : Predicate as a function argument
import java.util.List;
import java.util.function.Predicate;
class PredicateExampleWithFunction {
static void filterNumbers(List<Integer> numbers, Predicate<Integer> predicate) {
numbers.stream()
.filter(predicate)
.forEach(System.out::println);
}
public static void main(String[] args) {
List<Integer> numberList = List.of(1,2,3,4,5,6,7,8,9,10);
Predicate<Integer> isEven = (i) -> i % 2 == 0;
System.out.println("Even numbers:");
filterNumbers(numberList, isEven);
}
}
Example 7 : Predicate chaining example
import java.util.function.Predicate;
public class PredicateChainingExample {
public static void main(String[] args)
{
Predicate<Integer> greaterThanFive = (i) -> i > 5;
// Creating predicate
Predicate<Integer> lowerThanTen = (i) -> i < 10;
// Calling Predicate method
boolean result = greaterThanFive.and(lowerThanTen).negate().test(15);
System.out.println(result); // true
}
}
Benefits of Java 8 Predicate
- Streamlining Collection Operations: With Predicate, developers can filter collections based on specified criteria, simplifying code and improving readability.
- Enhanced Code Reusability: Predicate promotes code reuse by encapsulating filtering logic into reusable components, fostering modular and maintainable codebases.
- Flexible Composition: Predicate supports logical operations such as AND, OR, and NOT, enabling developers to compose complex conditions effortlessly.
Conclusion
Java 8 Predicate is a powerful tool in Java that can help developers to simplify their code and make it more readable. It is a functional interface that can be used to filter data based on certain conditions. By using Java Predicate, you can improve the readability of your code, make it more reusable and make it easier to test. If you are working with Java 8 or later, you should consider using Java Predicate in your code.
If you are preparing for Java 8 interviews then checkout this.
Good day! This is my first comment here so I just wanted to give a quick shout out and say I truly enjoy reading your blog posts.