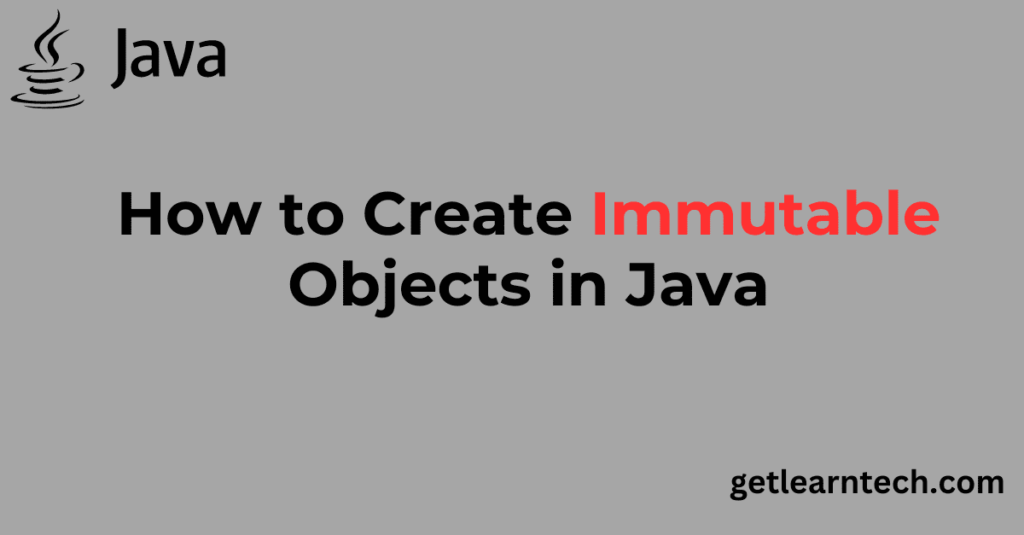
In the world of Java programming, immutability is a powerful concept that ensures the integrity and predictability of your code. Immutable objects, once created, cannot be modified, making them inherently thread-safe and resistant to bugs caused by unexpected changes. Its essential to know How to Create Immutable Objects in Java. In this article, we’ll delve deep into the principles of immutability and explore various techniques for creating immutable objects in Java.
You can find How to Create Immutable Objects in Java below.
Table of Contents
What are Immutable Objects?
Immutable objects are objects whose state (data) cannot be changed after they are created. Once an immutable object is created, its state remains constant throughout its lifetime. This property makes immutable objects inherently thread-safe and helps prevent bugs related to shared mutable state.
How to Create Immutable Objects in Java
1. Make the class final
To create an immutable class in Java, start by making the class final. This prevents the class from being subclassed and ensures that its state cannot be changed by any subclass.
public final class ImmutableObject {
// Class implementation
}
2. Make fields private and final
Next, make all fields in the class private and final. Private fields ensure encapsulation, while final fields prevent their values from being modified after initialization.
public final class ImmutableObject {
private final int id;
private final String name;
public ImmutableObject(int id, String name) {
this.id = id;
this.name = name;
}
// Getters for fields
}
3. Do not provide setter methods
Avoid providing setter methods in the class to prevent any modification of its state after creation. Instead, initialize the object’s state through the constructor.
4. Ensure mutable objects are not exposed
If your immutable class contains references to mutable objects (e.g., collections), make sure to return defensive copies or immutable versions of these objects to prevent external modification.
public final class ImmutableObject {
private final List<String> names;
public ImmutableObject(List<String> names) {
this.names = Collections.unmodifiableList(new ArrayList<>(names));
}
public List<String> getNames() {
return Collections.unmodifiableList(names);
}
}
Examples of immutable classes in Java
- String Class: The
String
class in Java is immutable, meaning once aString
object is created, its content cannot be changed. - Wrapper Classes: Wrapper classes like
Integer
,Long
,Double
, and others are also immutable in Java. Once an instance of these classes is created, their values cannot be modified.
Benefits of Immutable Objects
- Thread Safety: Immutable objects are inherently thread-safe since their state cannot be modified.
- Simplified Debugging: With immutable objects, you can avoid bugs related to shared mutable state.
- Caching: Immutable objects can be safely cached without worrying about their state changing.
- Security: Immutable objects are resistant to tampering and unintended modifications, reducing the risk of security vulnerabilities such as injection attacks or data corruption.
- Referential Transparency: Immutable objects exhibit referential transparency, meaning that the same input will always produce the same output, making the code more predictable and easier to reason about.
In conclusion, we hope you understood How to Create Immutable Objects in Java creating immutable objects in Java is a powerful technique that promotes code reliability and robustness. By following the principles outlined in this article and applying them to your Java classes, you can harness the benefits of immutability in your codebase. By leveraging immutability, you can write more robust, predictable, and thread-safe code, reducing the risk of bugs and improving the overall quality of your software. Whether you’re building multi-threaded applications or designing secure data structures, mastering the art of immutability is essential for Java developers at all levels.
Your writing style is fantastic – engaging, clear, and informative. Great work on this article!
Aprecio la profundidad de tu análisis comparativo entre diferentes modelos y teorías, lo que ofrece una comprensión más completa del tema.