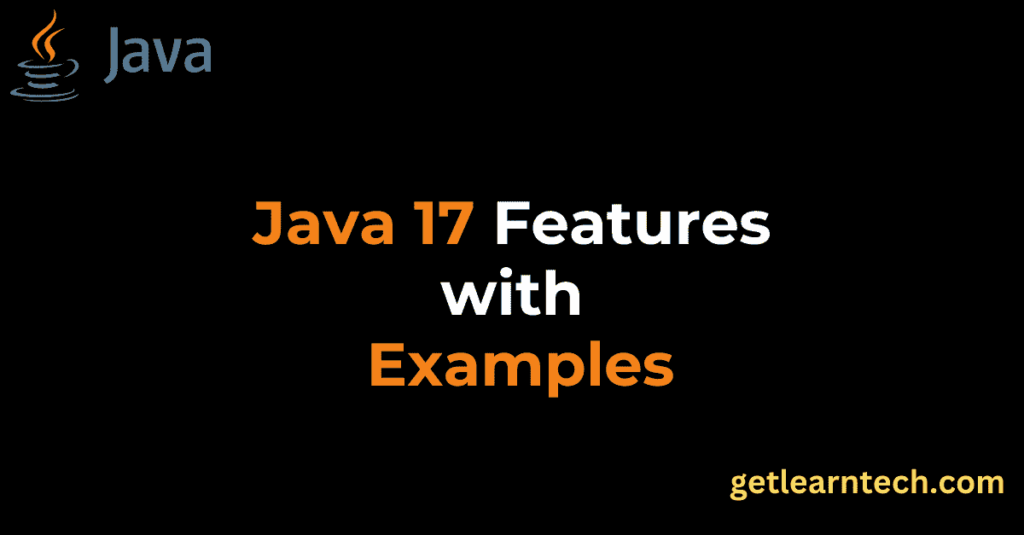
Java continues to evolve, introducing new features and enhancements that make development more efficient and powerful. Java 17, the latest long-term support (LTS) release, brings several significant updates that developers need to be aware of. In this article, we will explore Java 17 Features with Examples, provide practical examples, and outline some common interview questions related to these features.
Table of Contents
Java 17 Features with Examples
1. Sealed Classes (JEP 409)
Sealed classes allow developers to control which classes or interfaces can extend or implement them. This helps in designing a more robust and secure application architecture by limiting the inheritance hierarchy.
Sealed classes help enforce a strict class hierarchy, providing better control over inheritance.
Example :
public sealed class Animal permits Dog, Cat {
public abstract void makeSound();
}
public final class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Bark");
}
}
public final class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow");
}
}
Sealed Class Declaration
- Sealed Class: The
Animal
class is declared as a sealed class using thesealed
keyword. This keyword indicates that the class restricts which other classes or interfaces can extend or implement it. - Permitted Subclasses: The
permits
clause explicitly lists the classes that are allowed to extend the sealed classAnimal
. In this case, onlyDog
andCat
are permitted to extendAnimal
.
Abstract Method
- Abstract Method:
makeSound()
is an abstract method declared in the sealed classAnimal
. This method must be implemented by all non-abstract subclasses ofAnimal
. It defines a contract that any subclass must fulfill by providing its specific implementation.
Final Class Dog/Cat
- Final Class: The
Dog
class is declared asfinal
, meaning it cannot be subclassed further. This ensures that the class hierarchy remains limited and controlled. - Inheritance: The
Dog
class extends the sealed classAnimal
. AsDog
is listed in thepermits
clause ofAnimal
, it is allowed to inherit fromAnimal
. - Method Implementation: The
Dog
class provides its specific implementation of themakeSound()
method, which prints “Bark” to the console. This fulfills the contract defined by the abstract method inAnimal
.
Benefits of Sealed Classes:
- Enhanced Security: By controlling which classes can extend or implement the sealed class, developers can prevent unauthorized or unintended extensions. This reduces the risk of introducing bugs or vulnerabilities through unintended subclassing.
- Improved Maintainability: The explicit listing of permitted subclasses makes the class hierarchy clear and easy to understand. This helps in maintaining the code by providing a clear structure and reducing the likelihood of misuse.
- Compile-Time Verification: The compiler enforces the rules defined by the sealed class and its permitted subclasses. This means any attempt to create a subclass that is not listed in the
permits
clause will result in a compile-time error, ensuring that the intended design is followed strictly.
2. Pattern Matching for Switch (JEP 406)
This feature enhances the switch statement, allowing pattern matching to be used, making code more concise and readable.
Example :
public String typeTester(Object obj) {
return switch (obj) {
case String s -> "It's a string";
case Integer i -> "It's an integer";
default -> "Unknown type";
};
}
String result = switch (day) {
case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday" -> "Weekday";
case "Saturday", "Sunday" -> "Weekend";
default -> throw new IllegalArgumentException("Invalid day: " + day);
};
Benefits of Pattern Matching for Switch:
- Conciseness: Reduces boilerplate code by integrating type checks and casts directly into the switch statement.
- Readability: Makes the code more readable and easier to understand by eliminating redundant type-checking and casting code.
- Maintainability: Easier to maintain and extend, as adding new type patterns requires fewer lines of code and less manual casting.
3. New macOS Rendering Pipeline (JEP 382)
Java 17 introduces a new rendering pipeline for macOS, leveraging the Apple Metal API, which improves performance and reduces resource usage.
4. Foreign Function & Memory API (Incubator) (JEP 412)
This API enables Java programs to interoperate with native code and manage memory outside the Java heap, opening up new possibilities for high-performance applications.
Example :
try (ResourceScope scope = ResourceScope.newConfinedScope()) {
MemorySegment segment = MemorySegment.allocateNative(1024, scope);
// Use the allocated memory segment
}
5. Deprecation of the Applet API (JEP 398)
The Applet API is now deprecated, signaling an end to this outdated technology. Developers are encouraged to use modern web technologies instead.
Java 17 Features Interview Questions
What are sealed classes in Java 17 and why are they useful?
- Sealed classes restrict which other classes can extend them, providing better control over the class hierarchy and enhancing security.
How does pattern matching for switch improve code readability in Java 17?
- It allows type patterns to be used directly in switch cases, reducing boilerplate code and making conditional logic more concise and readable.
What improvements does the new macOS rendering pipeline in Java 17 offer?
- It uses the Apple Metal API for better performance and reduced resource usage, enhancing the rendering capabilities of Java applications on macOS.
Explain the Foreign Function & Memory API in Java 17.
- This API enables Java applications to call native functions and manage memory outside the Java heap, which is beneficial for performance-critical applications.
Why has the Applet API been deprecated in Java 17?
- The Applet API is outdated and insecure. Modern web technologies such as HTML5 and JavaScript are recommended alternatives.
Java 8 to Java 17 Features
Here’s a breakdown of key features introduced from Java 8 to Java 17:
Java 8 (March 2014):
- Lambda Expressions
- Stream API
- Default Methods
- New Date and Time API
- Optional Class
- Nashorn JavaScript Engine
- PermGen Space Removal
Java 9 (September 2017):
- Module System (Project Jigsaw)
- JShell (REPL)
- Improved Javadoc
- Process API Updates
- Collection Factory Methods
- Private Interface Methods
- HTTP/2 Client (Incubator)
Java 10 (March 2018):
- Local-Variable Type Inference (
var
) - Time-Based Release Versioning
- Garbage-Collector Interface
- Parallel Full GC for G1
Java 11 to Java 17 features
Java 11 (September 2018):
- HTTP Client API (Standardized)
- Local-Variable Syntax for Lambda Parameters
var
in Lambda Expressions- New
String
Methods - Unicode 10 Support
- Z Garbage Collector (ZGC)
- Flight Recorder
Java 12 (March 2019):
- Switch Expressions (Preview)
- Default CDS Archives
- JVM Constants API
- Shenandoah Garbage Collector (Experimental)
Java 13 (September 2019):
- Text Blocks (Preview)
- Dynamic CDS Archives
- ZGC: Uncommitting Unused Memory
- Reimplement the Legacy Socket API
Java 14 (March 2020):
- Switch Expressions (Standard)
- Records (Preview)
- Pattern Matching for
instanceof
(Preview) - Helpful NullPointerExceptions
Java 15 (September 2020):
- Text Blocks (Standard)
- Sealed Classes (Preview)
- Hidden Classes
- ZGC Improvements
Java 16 (March 2021):
- Records (Standard)
- Pattern Matching for
instanceof
(Standard) - Sealed Classes (Second Preview)
- Vector API (Incubator)
- Foreign Linker API (Incubator)
Java 17 (September 2021):
- Sealed Classes (Standard)
- Pattern Matching for Switch (Preview)
- New macOS Rendering Pipeline
- Foreign Function & Memory API (Incubator)
- Deprecation of the Applet API
Java 17 Features vs. Java 11
Java 17:
- Sealed Classes (Standard): Allows control over which classes can extend or implement them.
- Pattern Matching for Switch (Preview): Enhances switch statements with pattern matching capabilities.
- New macOS Rendering Pipeline: Utilizes Apple Metal API for better performance.
- Foreign Function & Memory API (Incubator): Enables Java to interoperate with native code and manage memory outside the Java heap.
- Deprecation of Applet API: The Applet API is marked for removal.
Java 11:
- HTTP Client API (Standardized): Provides a modern HTTP client.
- Local-Variable Syntax for Lambda Parameters: Use
var
in lambda expressions. - New
String
Methods: Includesrepeat
,lines
,strip
,isBlank
, etc. - Unicode 10 Support: Updated Unicode support.
- Z Garbage Collector (ZGC): A scalable low-latency garbage collector.
- Flight Recorder: A performance analysis tool integrated into the JDK.
If you are preparing for Java 8 interviews then you can checkout here.
To learn Java 8 concepts you can checkout here.
Conclusion
Java 17 introduces powerful features that enhance the language’s capabilities and improve developer productivity. From sealed classes and pattern matching for switch to a new macOS rendering pipeline and the Foreign Function & Memory API, Java 17 is packed with updates that every developer should explore. Understanding these features not only prepares you for modern Java development but also equips you with knowledge for technical interviews. We hope you liked Java 17 Features with Examples, please checkout our other articles.