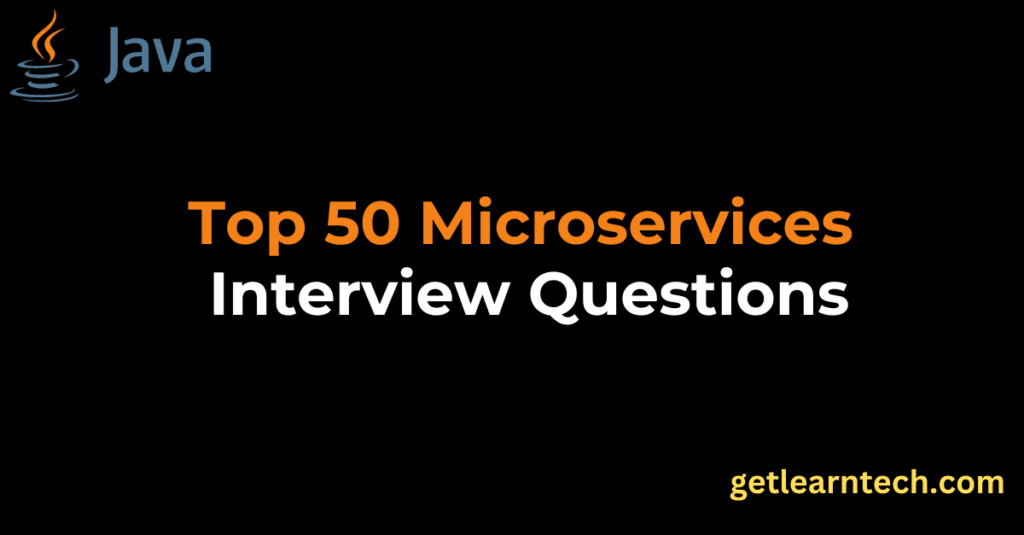
Microservices architecture has gained immense popularity in recent years due to its ability to create scalable, resilient, and independently deployable services. Whether you’re preparing for an interview as a beginner or an experienced professional, understanding microservices concepts is crucial. This article covers 50 microservices interview questions with detailed answers to help you ace your next interview.
Table of Contents
Microservices Interview Questions and Answers
1. What are Microservices?
Microservices, also known as the microservice architecture, is an architectural style that structures an application as a collection of loosely coupled services, each implementing a specific business capability. These services can be developed, deployed, and scaled independently.
2. How do Microservices differ from Monolithic Architecture?
In a monolithic architecture, the entire application is built as a single, unified unit. All functionalities are tightly integrated and run as a single process. In contrast, microservices architecture breaks down the application into smaller, independent services that can be developed and deployed separately.
3. What are the main benefits of using Microservices?
The benefits of microservices include:
- Scalability: Individual services can be scaled independently.
- Flexibility: Developers can use different technologies for different services.
- Resilience: Failure of one service doesn’t affect the entire system.
- Faster Time to Market: Teams can develop and deploy services independently.
4. What are some common challenges with Microservices?
Common challenges include:
- Complexity: Managing multiple services can be complex.
- Data Management: Handling data consistency across services.
- Deployment: Coordinating deployments of multiple services.
- Monitoring: Keeping track of many services requires advanced monitoring solutions.
5. What is the role of an API Gateway in Microservices?
An API Gateway acts as an entry point for clients to interact with the microservices. It handles request routing, composition, and protocol translation, and provides security, load balancing, and monitoring.
6. Can you explain the concept of “bounded context” in Microservices?
A bounded context is a design principle from Domain-Driven Design (DDD) that defines the boundaries within which a particular domain model applies. In microservices, each service corresponds to a bounded context, ensuring that the service has a clear and specific purpose.
7. How do Microservices communicate with each other?
Microservices communicate using lightweight protocols such as HTTP/HTTPS, REST, gRPC, or messaging queues (e.g., RabbitMQ, Kafka).
8. What is Service Discovery and why is it important?
Service Discovery is the process of automatically detecting devices and services on a network. In microservices, it helps services find each other dynamically without hardcoding the network locations, enabling easier scaling and failover.
9. What are some common patterns for inter-service communication in Microservices?
Common patterns include:
- Synchronous communication: Using REST or gRPC for direct service-to-service calls.
- Asynchronous communication: Using message brokers or event-driven architectures to decouple services.
10. Explain the Circuit Breaker pattern.
The Circuit Breaker pattern is used to detect failures and encapsulate the logic of preventing a failure from constantly recurring. It stops the service from making repeated requests when it’s likely to fail, thereby allowing the system to recover.
11. How do you handle data consistency in Microservices?
Data consistency can be handled using:
- Sagas: Distributed transactions pattern that manage a series of transactions that update each service and roll back if one fails.
- Eventual Consistency: Ensuring data consistency over time without immediate synchronization.
12. What are some strategies for deploying Microservices?
Strategies include:
- Blue-Green Deployment: Running two identical production environments.
- Canary Releases: Gradually rolling out the new version to a subset of users.
- Rolling Updates: Gradually updating instances of the application.
13. How do you implement security in Microservices?
Security can be implemented using:
- Authentication and Authorization: Using OAuth2, JWT tokens, etc.
- API Gateway: Managing security centrally.
- Service Mesh: For secure communication between services.
14. What is a Service Mesh and why is it used?
A Service Mesh is a dedicated infrastructure layer that manages service-to-service communication. It provides features like traffic management, security, and observability, allowing developers to focus on business logic.
15. How can you monitor Microservices?
Monitoring can be done using tools like Prometheus, Grafana, and ELK stack. Important aspects include logging, metrics, and distributed tracing (e.g., Jaeger, Zipkin).
16. What is the role of Docker in Microservices?
Docker simplifies the deployment and management of microservices by packaging them into containers, ensuring consistency across different environments.
17. How do you handle versioning in Microservices?
Versioning can be managed by:
- URI Versioning: Including version in the URL (e.g., /api/v1/resource).
- Header Versioning: Including version in the request header.
- Content Negotiation: Clients request a specific version via headers.
18. Explain the concept of “Event-Driven Architecture” in Microservices.
Event-Driven Architecture involves services communicating through events. An event is emitted when a state change occurs and other services can react to this event asynchronously.
19. What is the Two-Phase Commit (2PC) protocol?
The 2PC protocol is a type of atomic commitment protocol that ensures all participants in a distributed transaction either commit or roll back the transaction.
20. How can you ensure the resilience of Microservices?
Resilience can be ensured through:
- Retry Mechanisms: Automatically retrying failed requests.
- Bulkheads: Isolating failures within a service to prevent cascading failures.
- Circuit Breakers: Preventing constant retry on failures.
21. What is API Composition and how does it work?
API Composition is an approach where an API Gateway or another service aggregates data from multiple microservices and presents a unified response to the client.
22. What is eventual consistency and where is it used?
Eventual consistency is a consistency model where updates to a system are propagated to all nodes asynchronously. It is used in distributed systems where immediate consistency is not feasible.
23. How do you handle logging in a microservices environment?
Logging can be centralized using tools like ELK stack (Elasticsearch, Logstash, Kibana) or distributed tracing systems like Jaeger and Zipkin.
24. Explain the importance of container orchestration in Microservices.
Container orchestration manages the lifecycle of containers, including deployment, scaling, and networking. Kubernetes is a popular container orchestration tool.
25. What are sidecar patterns in Microservices?
Sidecar patterns involve deploying a helper service alongside the main service in a separate container. The sidecar can handle cross-cutting concerns like logging, configuration, and networking.
26. What is the Ambassador pattern?
The Ambassador pattern involves using a helper service that acts as an intermediary between the main service and the outside world. It handles common tasks such as authentication, logging, and monitoring.
27. How do you handle transactions in Microservices?
Transactions in microservices can be managed using:
- Sagas: Long-running transactions with compensating actions.
- Eventual Consistency: Ensuring data consistency over time.
28. What are CQRS and Event Sourcing?
- CQRS (Command Query Responsibility Segregation): Separates read and write operations.
- Event Sourcing: Persisting the state of a business entity as a sequence of events.
29. What is the Strangler Fig pattern?
The Strangler Fig pattern is a strategy to incrementally migrate a legacy system to a new system by replacing parts of the old system with new services.
30. How do you manage configuration in Microservices?
Configuration management can be handled using centralized configuration tools like Spring Cloud Config, Consul, or etcd.
31. What are some common tools for managing Microservices?
Common tools include:
- Docker: For containerization.
- Kubernetes: For orchestration.
- Istio: For service mesh.
- Consul: For service discovery and configuration.
32. How do you handle inter-service communication failures?
Handling communication failures can involve:
- Retries: Automatically retrying failed requests.
- Circuit Breakers: Preventing retries on persistent failures.
- Fallbacks: Providing alternative responses or actions.
33. What is a Distributed Tracing and why is it important?
Distributed tracing tracks a request as it travels through various services in a microservices architecture. It is important for debugging and performance monitoring.
34. What is API Gateway Pattern and its advantages?
The API Gateway Pattern involves using a single entry point for all client interactions. Advantages include:
- Simplified client communication.
- Load balancing and rate limiting.
- Centralized security and monitoring.
35. Explain the concept of “Domain-Driven Design” (DDD).
DDD is a design approach that focuses on modeling the domain according to the real-world business context, dividing the domain into bounded contexts and ensuring that each service encapsulates a specific part of the business logic.
36. What are some best practices for designing Microservices?
Best practices include:
- Defining clear service boundaries.
- Ensuring loose coupling and high cohesion.
- Automating testing and deployment.
- Implementing robust monitoring and logging.
37. How do you handle service versioning and backward compatibility?
Handling service versioning involves:
- Versioning APIs using URLs or headers.
- Maintaining backward compatibility by supporting old versions until clients migrate.
38. What is the importance of automation in Microservices?
Automation is crucial for:
- Continuous Integration/Continuous Deployment (CI/CD).
- Consistent environment setup and teardown.
- Automated scaling and management of services.
39. What are some popular frameworks for developing Microservices?
Popular frameworks include:
- Spring Boot: For Java.
- MicroProfile: For Java EE.
- Go-Micro: For Go.
- Node.js: For JavaScript.
40. How do you manage state in Microservices?
Managing state can be done using:
- Stateful services: Keeping state within the service.
- Stateless services: Externalizing state to databases or caching systems.
41. What is Service Orchestration?
Service Orchestration involves managing and coordinating multiple services to achieve a specific outcome. It ensures services work together in a defined workflow.
42. How do you handle schema changes in Microservices?
Schema changes can be managed using:
- Backward and forward-compatible schema.
- Database versioning tools like Flyway or Liquibase.
- Graceful migration strategies.
43. What is the concept of “API Gateway Offloading”?
API Gateway Offloading involves moving common tasks such as authentication, rate limiting, and logging from the microservices to the API Gateway.
44. How do you ensure high availability in Microservices?
High availability can be ensured by:
- Using redundant services.
- Load balancing.
- Implementing failover mechanisms.
45. What is a Distributed Cache and why is it used?
A distributed cache stores frequently accessed data in memory across multiple servers, improving performance and reducing database load. Examples include Redis and Memcached.
46. What are some data management patterns in Microservices?
Data management patterns include:
- Database per service.
- Event sourcing.
47. How do you manage dependencies in Microservices?
Dependency management involves:
- Using dependency injection frameworks.
- Managing library versions.
- Isolating dependencies to minimize impact.
48. What is an Anti-Corruption Layer (ACL)?
An ACL is a layer that translates communications between different systems, ensuring that changes in one system don’t corrupt the integrity of another.
49. What are the Twelve-Factor App principles?
The Twelve-Factor App principles are a set of best practices for building scalable and maintainable software-as-a-service (SaaS) applications. They include:
- Codebase: One codebase tracked in revision control.
- Dependencies: Explicitly declare and isolate dependencies.
- Config: Store config in the environment.
- Backing services: Treat backing services as attached resources.
50. How do you handle load balancing in Microservices?
Load balancing can be handled using:
- Client-side load balancing: Implemented in the client.
- Server-side load balancing: Using tools like Nginx or HAProxy.
- Service discovery: Automatically detecting service instances.
This comprehensive guide covers a wide range of microservices interview questions that cater to both beginners and experienced professionals. Understanding these concepts will not only help you in interviews but also in building robust microservices architectures.
If you are preparing Java interviews then do checkout here