Handling multiple if-else
statements in a Java project can quickly become cumbersome and hard to maintain. This guide will show you how to optimize and refactor such code using various strategies. These techniques will make your code cleaner, more efficient, and easier to manage. Let’s dive into several methods to replace extensive if-else
chains with more robust solutions.
If you are preparing Java interviews then checkout here.
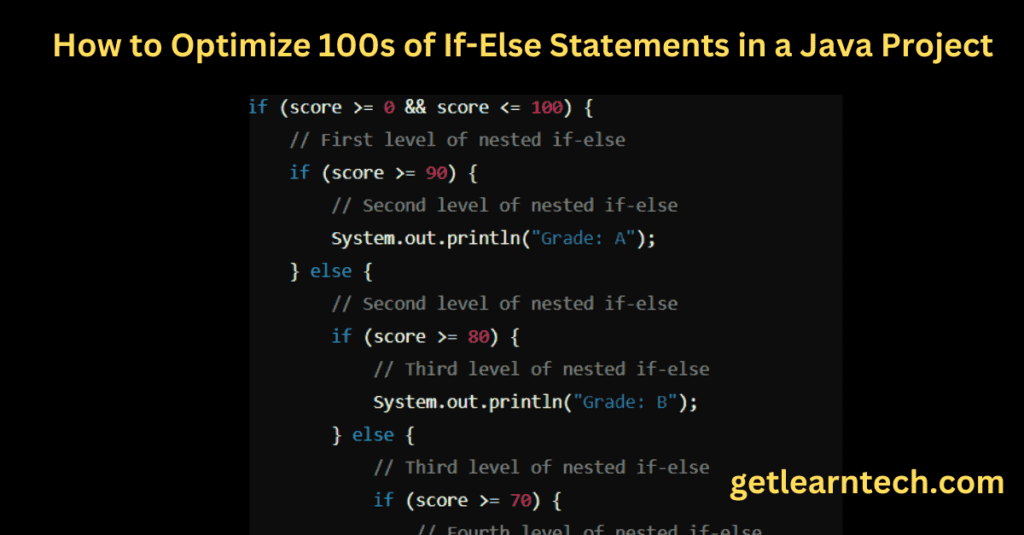
Table of Contents
Why Optimize If-Else Statements?
Before diving into the optimization techniques, it’s essential to understand why optimizing if-else
statements is beneficial:
- Readability: Long chains of
if-else
statements can be hard to read and understand. - Maintainability: Updating or debugging long
if-else
chains is error-prone and time-consuming. - Performance: While modern JVMs are quite efficient, extensive
if-else
chains can impact performance, especially if evaluated frequently.
Initial Example with Long If-Else Chain
Suppose you have a method that performs different actions based on a string input. Here is how it might look with a long if-else
chain:
public void processAction(String action) {
if (action.equals("action1")) {
performAction1();
} else if (action.equals("action2")) {
performAction2();
} else if (action.equals("action3")) {
performAction3();
} else {
performDefaultAction();
}
}
private void performAction1() {
System.out.println("Performing action 1");
}
private void performAction2() {
System.out.println("Performing action 2");
}
private void performAction3() {
System.out.println("Performing action 3");
}
private void performDefaultAction() {
System.out.println("Performing default action");
}
Optimization Techniques
1. Use a Map for Lookup
If your if-else
statements are used to handle different cases based on discrete values, a Map
can be a more efficient and cleaner solution.
Example
import java.util.HashMap;
import java.util.Map;
import java.util.function.Consumer;
public class ActionProcessor {
private static final Map<String, Consumer<ActionProcessor>> actions = new HashMap<>();
static {
actions.put("action1", ActionProcessor::performAction1);
actions.put("action2", ActionProcessor::performAction2);
actions.put("action3", ActionProcessor::performAction3);
}
public void processAction(String action) {
actions.getOrDefault(action, ActionProcessor::performDefaultAction).accept(this);
}
private void performAction1() {
System.out.println("Performing action 1");
}
private void performAction2() {
System.out.println("Performing action 2");
}
private void performAction3() {
System.out.println("Performing action 3");
}
private void performDefaultAction() {
System.out.println("Performing default action");
}
public static void main(String[] args) {
ActionProcessor processor = new ActionProcessor();
processor.processAction("action1"); // Output: Performing action 1
processor.processAction("action3"); // Output: Performing action 3
processor.processAction("unknown"); // Output: Performing default action
}
}
2. Use Enum with Strategy Pattern
For handling a fixed set of operations, using an enum
with a strategy pattern can be very effective.
Example
public enum Action {
ACTION1 {
@Override
void perform() {
System.out.println("Performing action 1");
}
},
ACTION2 {
@Override
void perform() {
System.out.println("Performing action 2");
}
},
ACTION3 {
@Override
void perform() {
System.out.println("Performing action 3");
}
},
DEFAULT {
@Override
void perform() {
System.out.println("Performing default action");
}
};
abstract void perform();
}
public class ActionProcessor {
public void processAction(String action) {
try {
Action.valueOf(action.toUpperCase()).perform();
} catch (IllegalArgumentException e) {
Action.DEFAULT.perform();
}
}
public static void main(String[] args) {
ActionProcessor processor = new ActionProcessor();
processor.processAction("ACTION1"); // Output: Performing action 1
processor.processAction("ACTION3"); // Output: Performing action 3
processor.processAction("UNKNOWN"); // Output: Performing default action
}
}
3. Use Polymorphism
If your if-else
statements are selecting different behaviors based on the type, polymorphism can replace conditionals with class hierarchies.
Example
abstract class Action {
abstract void perform();
}
class Action1 extends Action {
@Override
void perform() {
System.out.println("Performing action 1");
}
}
class Action2 extends Action {
@Override
void perform() {
System.out.println("Performing action 2");
}
}
class Action3 extends Action {
@Override
void perform() {
System.out.println("Performing action 3");
}
}
class DefaultAction extends Action {
@Override
void perform() {
System.out.println("Performing default action");
}
}
public class ActionProcessor {
private static final Map<String, Action> actions = new HashMap<>();
static {
actions.put("action1", new Action1());
actions.put("action2", new Action2());
actions.put("action3", new Action3());
}
public void processAction(String action) {
actions.getOrDefault(action, new DefaultAction()).perform();
}
public static void main(String[] args) {
ActionProcessor processor = new ActionProcessor();
processor.processAction("action1"); // Output: Performing action 1
processor.processAction("action3"); // Output: Performing action 3
processor.processAction("unknown"); // Output: Performing default action
}
}
4. Using Function Objects
Java 8 introduced functional programming constructs that can help streamline your code.
Example
import java.util.HashMap;
import java.util.Map;
import java.util.function.Consumer;
public class ActionProcessor {
private static final Map<String, Consumer<ActionProcessor>> actions = new HashMap<>();
static {
actions.put("action1", ActionProcessor::performAction1);
actions.put("action2", ActionProcessor::performAction2);
actions.put("action3", ActionProcessor::performAction3);
}
public void processAction(String action) {
actions.getOrDefault(action, ActionProcessor::performDefaultAction).accept(this);
}
private void performAction1() {
System.out.println("Performing action 1");
}
private void performAction2() {
System.out.println("Performing action 2");
}
private void performAction3() {
System.out.println("Performing action 3");
}
private void performDefaultAction() {
System.out.println("Performing default action");
}
public static void main(String[] args) {
ActionProcessor processor = new ActionProcessor();
processor.processAction("action1"); // Output: Performing action 1
processor.processAction("action3"); // Output: Performing action 3
processor.processAction("unknown"); // Output: Performing default action
}
}
5. State Pattern
If your if-else
chain is managing state transitions, consider using the state pattern to encapsulate state-specific behavior.
Example
interface State {
void handle();
}
class StateA implements State {
@Override
public void handle() {
System.out.println("Handling state A");
}
}
class StateB implements State {
@Override
public void handle() {
System.out.println("Handling state B");
}
}
class StateC implements State {
@Override
public void handle() {
System.out.println("Handling state C");
}
}
class Context {
private State state;
public void setState(State state) {
this.state = state;
}
public void request() {
state.handle();
}
}
public class StatePatternExample {
public static void main(String[] args) {
Context context = new Context();
context.setState(new StateA());
context.request(); // Output: Handling state A
context.setState(new StateB());
context.request(); // Output: Handling state B
context.setState(new StateC());
context.request(); // Output: Handling state C
}
}
Conclusion
Optimizing 1000 if-else
statements in a Java project might seem daunting, but with the right techniques, you can significantly improve the readability, maintainability, and performance of your code. By using maps for lookups, enums with strategy patterns, polymorphism, function objects, and the state pattern, you can transform cumbersome conditionals into clean, efficient, and scalable solutions. Start by identifying the most suitable approach for your specific scenario and incrementally refactor your code. Happy coding!
By implementing these strategies, you’ll not only make your code more efficient but also easier to read and maintain. This will ultimately lead to a more robust and scalable Java application.