Binary search is a highly efficient algorithm for finding an item from a sorted list of items. It works by repeatedly dividing in half the portion of the list that could contain the item until you’ve narrowed down the possible locations to just one. In this article, we’ll dive into binary search in Java, exploring both recursive and iterative implementations, and providing example code snippets to aid understanding.
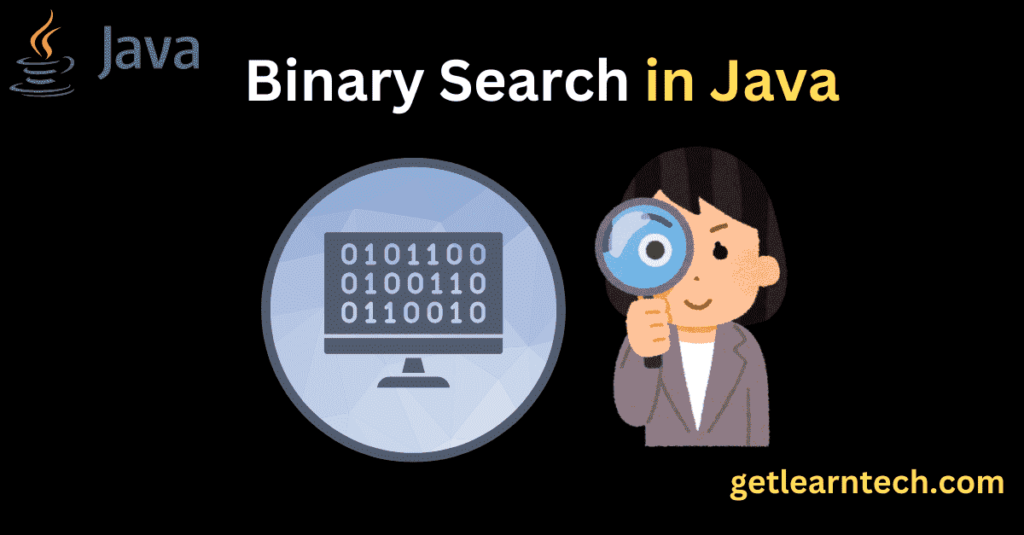
Table of Contents
What is Binary Search?
Binary search is an algorithm used to find the position of a target value within a sorted array. The basic idea is to divide the array in half, compare the target value with the middle element, and then repeat the process in the appropriate half.
Binary Search in Java
Java provides various ways to implement binary search. We will cover both recursive and iterative approaches.
Recursive Binary Search in Java
In a recursive binary search, the algorithm calls itself with a smaller portion of the array. Here’s the implementation:
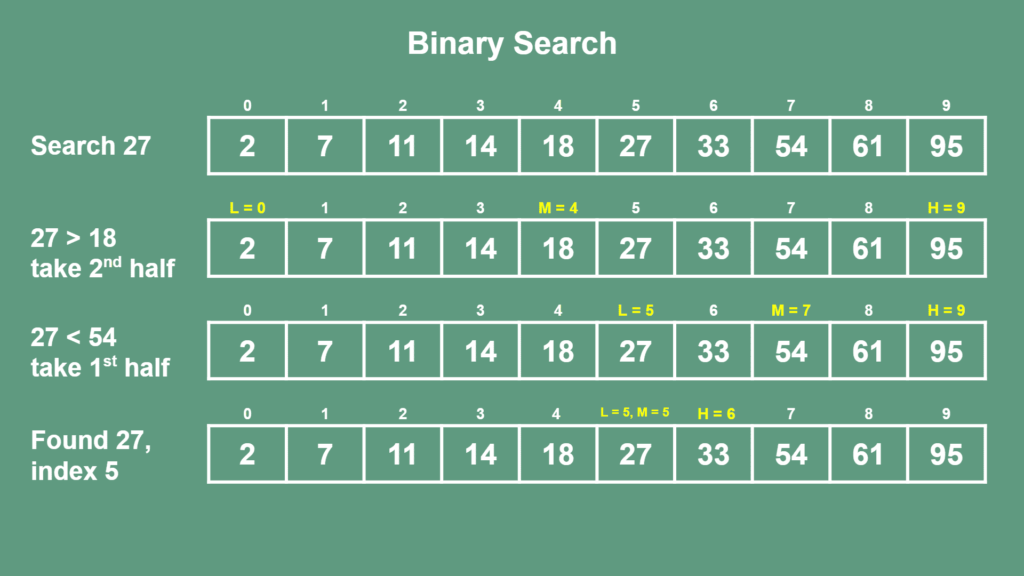
public class BinarySearch {
public static int recursiveBinarySearch(int[] array, int target, int low, int high) {
if (low > high) {
return -1; // Target not found
}
int mid = low + (high - low) / 2;
if (array[mid] == target) {
return mid;
} else if (array[mid] > target) {
return recursiveBinarySearch(array, target, low, mid - 1);
} else {
return recursiveBinarySearch(array, target, mid + 1, high);
}
}
public static void main(String[] args) {
int[] array = {2, 7, 11, 14, 18, 27, 33, 54, 61, 95};
int target = 27;
int result = recursiveBinarySearch(array, target, 0, array.length - 1);
System.out.println("Element found at index: " + result);
}
}
Explanation:
- The
recursiveBinarySearch
method takes an array, a target value, and the low and high indices as parameters. - If
low
is greater thanhigh
, the target is not found, and the method returns -1. - The
mid
index is calculated as the average oflow
andhigh
. - If the middle element is equal to the target, the method returns the
mid
index. - If the middle element is greater than the target, the method recursively searches the left half of the array.
- If the middle element is less than the target, the method recursively searches the right half of the array.
Iterative Binary Search in Java
The iterative approach avoids the overhead of recursive calls and is generally more space-efficient. Here’s how you can implement it:
public class BinarySearch {
public static int iterativeBinarySearch(int[] array, int target) {
int low = 0;
int high = array.length - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
if (array[mid] == target) {
return mid;
} else if (array[mid] > target) {
high = mid - 1;
} else {
low = mid + 1;
}
}
return -1; // Target not found
}
public static void main(String[] args) {
int[] array = {1, 3, 5, 7, 9, 11};
int target = 7;
int result = iterativeBinarySearch(array, target);
System.out.println("Element found at index: " + result);
}
}
Explanation:
- The
iterativeBinarySearch
method initializeslow
andhigh
to the start and end of the array, respectively. - It enters a while loop that continues as long as
low
is less than or equal tohigh
. - The
mid
index is calculated within the loop. - If the middle element is equal to the target, the method returns the
mid
index. - If the middle element is greater than the target,
high
is updated tomid - 1
. - If the middle element is less than the target,
low
is updated tomid + 1
. - If the loop ends without finding the target, the method returns -1.
Arrays Binary Search in Java
Java also provides a built-in method for binary search in the Arrays
class. Here’s an example:
import java.util.Arrays;
public class BinarySearchExample {
public static void main(String[] args) {
int[] array = {1, 3, 5, 7, 9, 11};
int target = 7;
int result = Arrays.binarySearch(array, target);
System.out.println("Element found at index: " + result);
}
}
Explanation:
- The
Arrays.binarySearch
method simplifies the process of implementing binary search. - It takes the array and the target value as parameters and returns the index of the target value if found, or a negative value if the target is not found.
Conclusion
Binary search is an essential algorithm in computer science, offering a time complexity of O(log n), which makes it highly efficient for searching in sorted arrays. In Java, you can implement binary search using both recursive and iterative methods, or utilize the built-in method provided by the Arrays
class.
By mastering these techniques, you can enhance your problem-solving skills and be better prepared for technical interviews. Whether you use recursive binary search in Java, iterative binary search, or the built-in method, understanding the underlying principles will help you write efficient and effective code.