Java developers often encounter various exceptions and errors during development. Two of the most common are ClassNotFoundException
and NoClassDefFoundError
. Understanding the differences between these can help in effectively diagnosing and fixing issues in Java applications. This article will explore these two errors, their causes, and how to resolve them.
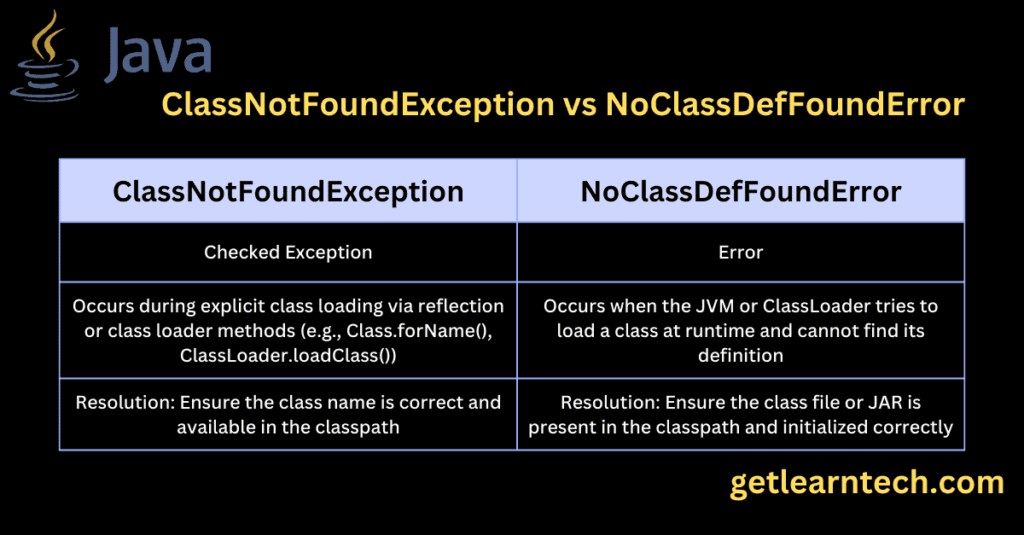
Table of Contents
ClassNotFoundException vs NoClassDefFoundError in Java
What is ClassNotFoundException?
ClassNotFoundException
is a checked exception that occurs when an application tries to load a class at runtime using methods like Class.forName()
, ClassLoader.loadClass()
, or ClassLoader.findSystemClass()
and the class is not found in the classpath. This exception must be caught and handled explicitly in the code.
Common Causes
- Classpath Issues: The specified class is not present in the classpath.
- Typographical Errors: Incorrect class name or package name.
- Class Loader Issues: The class loader being used does not have access to the class.
try {
Class.forName("com.example.MyClass");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
In this example, ClassNotFoundException
will be thrown if com.example.MyClass
is not found in the classpath.
What is NoClassDefFoundError?
NoClassDefFoundError
is an error that occurs when the Java Virtual Machine (JVM) or a ClassLoader
instance tries to load a class and the definition of the class (i.e., the .class
file) is not found. This error can occur even if the class was present at compile-time but is missing at runtime.
Common Causes
- The class was present at compile time, but not at runtime.
- The class was removed from the classpath after compilation.
- The class is available at compile time, but not during runtime due to a different classpath or class version conflicts.
- Version mismatch between the compiled classes and the runtime environment, especially if using different versions of dependencies or the JDK.
- The class file or JAR might be corrupted, making it unreadable.
public class Main {
public static void main(String[] args) {
MyClass myObject = new MyClass(); // Throws NoClassDefFoundError if MyClass is not found at runtime
}
}
In this example, NoClassDefFoundError
will be thrown if MyClass
was available at compile time but is missing at runtime.
ClassNotFoundException vs. NoClassDefFoundError Differences
Sure, here is the table with the differences between ClassNotFoundException
and NoClassDefFoundError
:
Aspect | ClassNotFoundException | NoClassDefFoundError |
---|---|---|
Type | Checked Exception | Error |
When It Occurs | During explicit class loading via reflection or class loader methods (e.g., Class.forName() , ClassLoader.loadClass() ) | When the JVM or ClassLoader tries to load a class at runtime and cannot find its definition |
Common Cause | Class name provided is not found in the classpath | Class was present during compile time but missing at runtime or failed during initialization |
Handling | Must be caught or declared in a throws clause | Typically not caught; indicates a more serious problem |
Example Scenario | Using Class.forName("com.example.MyClass") and the class is not found | Instantiating a class that was available during compilation but missing during runtime |
Typical Resolution | Ensure the class name is correct and available in the classpath | Ensure the class file or JAR is present in the classpath and initialized correctly |
Inheritance | Extends Exception | Extends Error |
Severity | Less severe, can often be resolved by correcting classpath issues or class names | More severe, often indicates a configuration or deployment issue |
Common Scenarios of NoClassDefFoundError in Java
NoClassDefFoundError in Java
In Java, NoClassDefFoundError
often appears when there is a discrepancy between compile-time and runtime classpaths. For instance, if a class was available during compilation but is missing during execution, the JVM throws a NoClassDefFoundError
.
Exception in Thread Main: Java.lang.NoClassDefFoundError
The error message “Exception in thread main: java.lang.NoClassDefFoundError” indicates that the main method of the application fails to start because a required class is missing. This can occur due to various reasons such as a missing JAR file, incorrect classpath settings, or a corrupted class file.
Specific Cases of ClassNotFoundException and NoClassDefFoundError
NoClassDefFoundError org.apache.commons.logging.LogFactory
A common example is the NoClassDefFoundError
related to the Apache Commons Logging library. If your application depends on org.apache.commons.logging.LogFactory
and this class is not found at runtime, it indicates that the commons-logging JAR file is missing from your classpath. Ensure that all required dependencies are included in your build configuration, such as Maven or Gradle, to prevent such errors.
Java.lang.ClassNotFoundException org.apache.jsp.index_jsp
ClassNotFoundException
can also occur in web applications, such as when the class org.apache.jsp.index_jsp
is not found. This typically happens in JSP (JavaServer Pages) applications when the JSP files are not properly compiled or the compiled classes are not available in the expected location.
Error Occurred During Initialization of VM: java/lang/NoClassDefFoundError: java/lang/Object
A critical instance of NoClassDefFoundError
is “Error occurred during initialization of VM: java/lang/NoClassDefFoundError: java/lang/Object.” This suggests that the JVM cannot find the fundamental class java.lang.Object
, which is essential for the Java runtime environment. Such an error usually indicates a severely corrupted JRE installation or misconfigured environment.
Troubleshooting ClassNotFoundException and NoClassDefFoundError
Here are steps to diagnose and fix these errors:
For ClassNotFoundException
- Check the Classpath: Ensure that the classpath includes all necessary JAR files and directories.
- Verify Dynamic Class Loading: Ensure that the class names passed to methods like
Class.forName()
are correct. - Inspect Build Configuration: For build tools like Maven or Gradle, verify that dependencies are correctly specified and included in the build.
For NoClassDefFoundError
- Check the Classpath: Ensure that all required JAR files and directories are included in the classpath.
- Verify Dependency Inclusion: Ensure all dependencies are properly included in your build tool configuration.
- Inspect Runtime Environment: Ensure the runtime environment is correctly configured and that no essential files are missing.
- Check for Corruption: Verify that JAR files and the JRE installation are not corrupted. Reinstalling might be necessary if corruption is detected.
Conclusion
Both ClassNotFoundException
and NoClassDefFoundError
are common issues in Java development that stem from classpath misconfigurations or missing class definitions. By understanding their causes and differences, developers can more effectively troubleshoot and resolve these errors. Ensuring proper configuration of the development and runtime environments, along with diligent dependency management, can prevent many occurrences of these errors. We hope you understood difference between ClassNotFoundException vs NoClassDefFoundError in Java.
Some more common issues which you can encounter during Java development.
Fix for java.lang.ClassNotFoundException: com.mysql.cj.jdbc.Driver