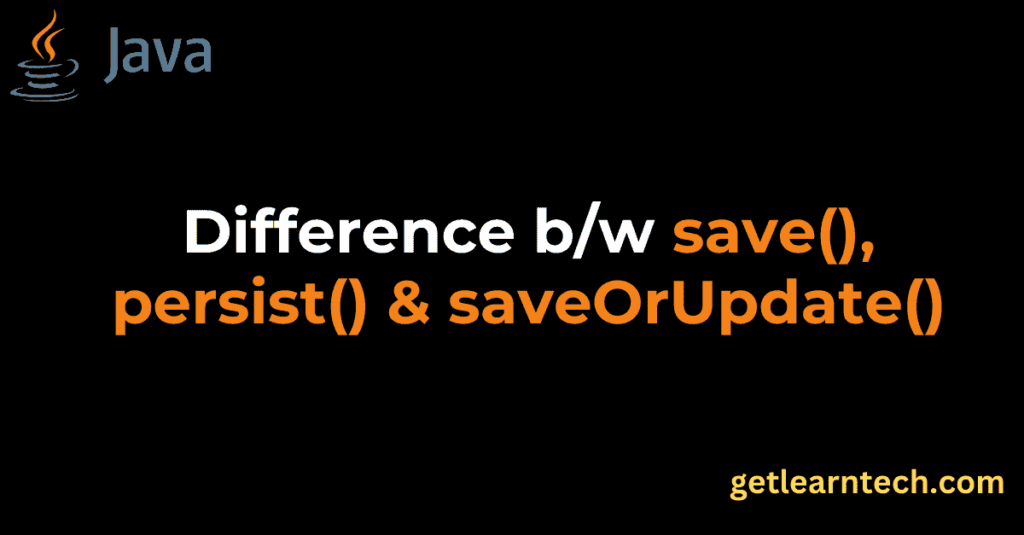
Table of Contents
When working with Hibernate, understanding the differences between save
, persist
, and saveOrUpdate
is crucial for efficient and effective data management. These methods might seem similar at first glance, but they serve distinct purposes and behave differently under various circumstances. In this article, we’ll explore the key differences and use cases for each method, helping you make informed decisions when managing entities in your Hibernate application.
Differences between save
(), persist
(), and saveOrUpdate
()
save()
Method
The save()
method is one of the most commonly used methods in Hibernate. It’s part of the Session
interface and is used to insert a new record into the database.
Key Characteristics:
- Inserts a new record: The
save()
method is designed to insert a new entity into the database. It generates a new identifier and ensures that the entity is saved as a new row. - Returns the generated identifier: After saving the entity, the
save()
method returns the generated identifier (usually the primary key) of the saved entity. - Immediate execution: When
save()
is called, Hibernate immediately executes anINSERT
SQL statement. - Not part of the JPA standard: The
save()
method is specific to Hibernate and is not part of the Java Persistence API (JPA) standard.
Use Case:
- Use
save()
when you are sure that the entity is new and needs to be inserted into the database.
Example:
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
Long id = (Long) session.save(entity);
tx.commit();
session.close();
persist()
Method
The persist()
method is also used to insert a new entity into the database, but it has some differences compared to save()
.
Key Characteristics:
- Part of the JPA standard: Unlike
save()
,persist()
is a JPA-compliant method and can be used in a JPA environment. - Does not return an identifier: The
persist()
method does not return the generated identifier after inserting the entity. - Delays execution until flush: The
persist()
method doesn’t execute anINSERT
statement immediately. The actual SQL execution is delayed until theflush()
operation is triggered. - Works within a transaction: Since
persist()
is often used in JPA, it works seamlessly within the context of a transaction.
Use Case:
- Use
persist()
when you are working in a JPA-compliant environment and do not need to immediately obtain the generated identifier.
Example:
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
em.persist(entity);
em.getTransaction().commit();
em.close();
saveOrUpdate()
Method
The saveOrUpdate()
method is a more versatile method that can handle both new and existing entities.
Key Characteristics:
- Inserts or updates: If the entity does not exist in the database (determined by the identifier),
saveOrUpdate()
will insert it. If it does exist, the method will update the existing record. - Determines entity state: Hibernate automatically determines whether the entity is transient (new) or persistent (existing) and performs the appropriate action.
- Immediate execution: Similar to
save()
, thesaveOrUpdate()
method triggers an immediate SQL execution for either anINSERT
or anUPDATE
statement.
Use Case:
- Use
saveOrUpdate()
when you need a method that can handle both creating new entities and updating existing ones.
Example:
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
session.saveOrUpdate(entity);
tx.commit();
session.close();
Key Differences at a Glance
Feature | save() | persist() | saveOrUpdate() |
---|---|---|---|
JPA Standard | No | Yes | No |
Returns Identifier | Yes | No | No |
Immediate Execution | Yes | No (executes on flush) | Yes |
Operation Type | Insert | Insert | Insert or Update |
Use Case | Insert new entities | Insert new entities in JPA | Handle both new and existing entities |
Conclusion
Choosing the right method—save()
, persist()
, or saveOrUpdate()
—depends on your specific requirements. If you need a JPA-compliant method, persist()
is the way to go. For immediate inserts with a returned identifier, save()
is ideal. And if you need a method that handles both inserts and updates, saveOrUpdate()
provides the flexibility you need. Understanding these differences can help you optimize your Hibernate applications and manage your data more effectively.
By mastering these methods, you’ll be better equipped to handle various scenarios in your Hibernate projects, leading to more robust and maintainable code.
If you are preparing Java interviews you can checkout here.
I’ve been absent for some time, but now I remember why I used to love this blog. Thank you, I’ll try and check back more often. How frequently you update your site?