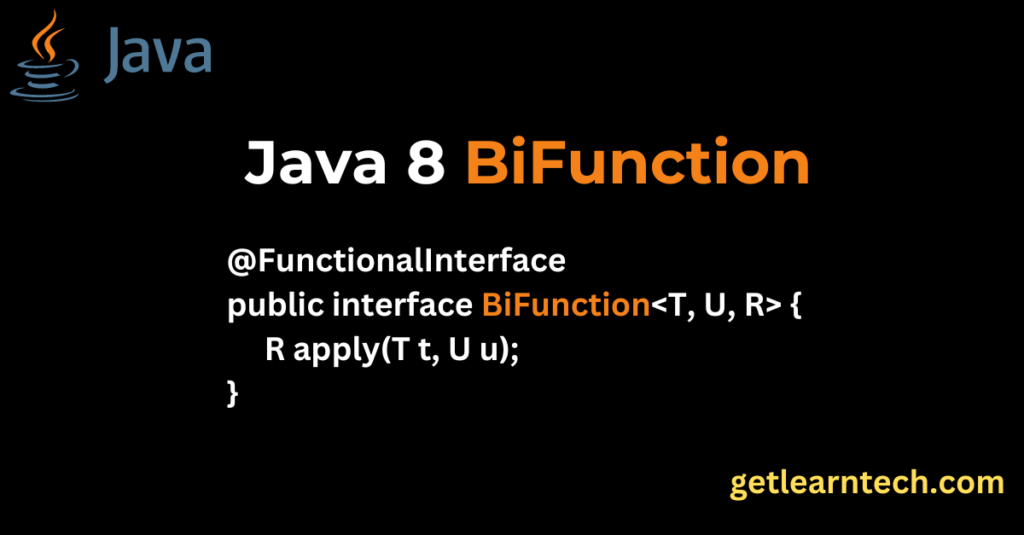
Table of Contents
Introduction
Java 8 introduced a new functional interface called BiFunction, which is a part of the java.util.function package. This interface represents a function that takes two arguments and produces a result. In this article, we will explore the BiFunction interface, its methods, and how to use it in Java programs.
What is BiFunction in Java ?
Java BiFunction interface is a functional interface that represents a function with two arguments and a result. It is similar to the Function interface, which has only one argument, but BiFunction has two. The BiFunction interface is used to represent functions that take two arguments and return a result.
Here’s the definition of the BiFunction interface:
@FunctionalInterface
public interface BiFunction<T, U, R> {
R apply(T t, U u);
default <V> BiFunction<T, U, V> andThen(Function<? super R, ? extends V> after) {
Objects.requireNonNull(after);
return (t, u) -> after.apply(apply(t, u));
}
}
The Java BiFunction interface has a single abstract method called apply()
, which takes two arguments of types T
and U
and returns a result of type R
. The andThen()
method is a default method that allows chaining of functions.
Using BiFunction:
To use the Java BiFunction interface in program, you need to create an instance of the class and then use its apply()
method to apply the function to two arguments.
Java BiFunction Examples :
Example 1 :
Below is the Java BiFunction example:
In this example, we define a BiFunction called add
that takes two integers and returns their sum. We then use the apply()
method to add two numbers and print the result.
We also define a BiFunction called concat
that takes two strings and returns their concatenation. We then use the apply()
method to concatenate two strings and print the result.
import java.util.function.BiFunction;
public class BiFunctionExample {
public static void main(String[] args) {
BiFunction<Integer, Integer, Integer> add = (x, y) -> x + y;
int result = add.apply(5, 3);
System.out.println(result);
BiFunction<String, String, String> concat = (x, y) -> x.concat(y);
String result2 = concat.apply("Hello", " World");
System.out.println(result2);
}
}
Example 2 :
To use BiFunction in streams, we can leverage the map()
function along with the BiFunction to transform elements in the stream. Here’s an example demonstrating the use of BiFunction in streams:
In this example, we have a stream of integers numbers
from 1 to 5. We define a BiFunction called add
that adds two integers. We then use the map()
function on the stream to apply the BiFunction and add 10 to each element in the stream. Finally, we print the modified numbers using forEach()
.
import java.util.stream.Stream;
import java.util.function.BiFunction;
public class BiFunctionInStreamsExample {
public static void main(String[] args) {
Stream<Integer> numbers = Stream.of(1, 2, 3, 4, 5);
BiFunction<Integer, Integer, Integer> add = (x, y) -> x + y;
// Using BiFunction in a stream to add 10 to each element
Stream<Integer> modifiedNumbers = numbers.map(num -> add.apply(num, 10));
modifiedNumbers.forEach(System.out::println);
}
}
FAQs
What is the difference between BiFunction and binary operator?
BiFunction and BinaryOperator are both functional interfaces in Java that represent functions with two arguments and a result. However, they differ in their return types and the types of their arguments.
Java BiFunction is a functional interface that takes two arguments of any type and returns a result of any type. It is defined as BiFunction<T, U, R>
, where T
and U
are the types of the input arguments and R
is the type of the result.
BinaryOperator, on the other hand, is a specialization of BiFunction that takes two arguments of the same type and returns a result of the same type. It is defined as BinaryOperator<T>
, where T
is the type of the input arguments and the result.
What is the difference between function and BiFunction in Java?
BiFunction<T, U, R> takes two arguments of any type and returns a result of any type, whereas Function<T, R> takes one argument of type T and returns a result of type R.
Conclusion
Java BiFunction interface is a powerful tool in Java functional programming that allows you to represent functions with two arguments and a result. By using the BiFunction interface, you can write more concise and expressive code that is easier to read and maintain. The Java BiFunction interface provides a more elegant solution to the common problem of handling functions with multiple arguments in Java programs.