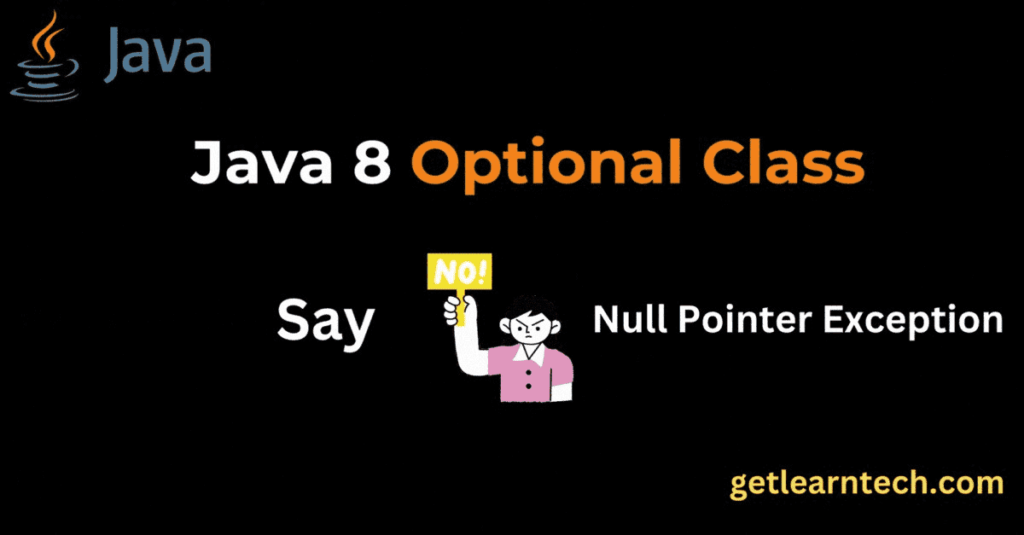
Table of Contents
Introduction
In Java programming, handling null values has always been a challenge. With Java 8 Optional class emerged as a powerful tool to address this issue. In this article, we’ll explore the concept of Optionals in Java, understanding their significance and learn to manage null values efficiently.
Understanding Optionals
An Optional in Java represents a container object that may or may not contain a non-null value. It provides a cleaner and safer alternative to dealing with null values, offering methods for easy retrieval, manipulation, and transformation of potentially absent values. The class is part of the java.util package and it is used to represent a value that may or may not be present.
Creating Optionals
Optionals in Java can be created using factory methods provided by the Optional class. These methods include of
, ofNullable
, and empty
, each serving a specific purpose:
Optional.of(value)
: Creates an Optional containing the specified non-null value.Optional.ofNullable(value)
: Creates an Optional containing the specified value if it’s non-null, otherwise returns an empty Optional.Optional.empty()
: Returns an empty Optional instance.
Example :
Optional<String> optionalName = Optional.of("John");
Optional<String> optionalNull = Optional.ofNullable(null);
Optional<String> optionalEmpty = Optional.empty();
In this example, optionalName
contains a non-null value (“John”), optionalNull
is empty due to the null input, and optionalEmpty
is an empty Optional.
Here are some of the most commonly used methods of the Optional class:
isPresent()
: Returns true if the Optional object contains a non-null value.get()
: Returns the non-null value contained in the Optional object.ifPresent(Consumer<? super T> consumer)
: Executes the given consumer action if the Optional object contains a non-null value.orElse(T other)
: Returns the non-null value contained in the Optional object, or the given default value if the Optional object is empty.orElseGet(Supplier<? extends T> supplier)
: Returns the non-null value contained in the Optional object, or the value returned by the given supplier if the Optional object is empty.orElseThrow(Supplier<? extends X> exceptionSupplier)
: Returns the non-null value contained in the Optional object, or throws the given exception if the Optional object is empty.
Here’s an example of how to use the Optional class in a Java program:
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
// create an Optional object with a non-null value
Optional<String> optional = Optional.of("Hello World!");
// check if the Optional object contains a non-null value
if (optional.isPresent()) {
// get the non-null value
String value = optional.get();
System.out.println(value);
}
// execute a consumer action if the Optional object contains a non-null value
optional.ifPresent(s -> System.out.println(s.toUpperCase()));
// create an Optional object with a null value
Optional<String> optionalNull = Optional.ofNullable(null);
// use the orElse method to get a default value if the Optional object is empty
String defaultValue = optionalNull.orElse("Default Value");
System.out.println(defaultValue);
// use the orElseGet method to get a default value if the Optional object is empty
String defaultValueGet = optionalNull.orElseGet(() -> "Default Value Get");
System.out.println(defaultValueGet);
// use the orElseThrow method to get a default value if the Optional object is empty
try {
String defaultValueThrow = optionalNull.orElseThrow(() -> new Exception("Optional is empty"));
System.out.println(defaultValueThrow);
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
Benefits of Optionals in Java
- Null-Safe Operations: Optionals facilitate null-safe operations, reducing the risk of NullPointerExceptions and improving code reliability.
- Expressive Error Handling: Optionals encourage explicit handling of absent values, leading to more readable and maintainable code.
- Functional Programming Support: Optionals seamlessly integrate with Java’s functional programming features, enabling concise and elegant solutions to common programming problems.
FAQs
What is Java Optional For?
Optionals in Java serves primarily as a tool for handling null values more effectively and safely. It addresses the challenges associated with null references, such as NullPointerExceptions, by providing a clear and expressive way to represent values that may or may not be present.
What is Optional in Java Streams?
In Java Streams, the Optional class is used to represent the possibility of encountering an empty result during stream operations. It’s returned by certain stream methods like findFirst()
or max()
to handle cases where no element is found.
When should you use Optionals ?
Use Optionals in Java when handling cases where a method may or may not produce a result, enforcing explicit handling of null cases, or dealing with Java Streams and the possibility of empty results.
What is the difference between Optional and List in Java ?
Optional: Represents an optional value that may or may not be present. It is used to avoid null references and indicate the possibility of absence. Optional is not a collection it does not support the ‘List’ type, so you cannot use ‘Optional<List>’ in Java. Optional is not a collection and can hold at most one value.
What are Optionals in Java ?
Optionals in Java are a container type introduced in Java 8 that represents an optional value that may or may not be present. They are used to eliminate the problem of null references by explicitly indicating the presence or absence of a value. Optionals encourage safer and more expressive code by forcing developers to handle null cases explicitly.
If you are preparing for Java 8 interviews then checkout this.
Conclusion
The Optionals in Java is a container object that may or may not contain a non-null value. It is designed to provide a more elegant solution to the common problem of null values in Java programs. By using the Optional class, you can write more robust and maintainable code that is less prone to NullPointerExceptions. The class provides several methods that allow you to check if the Optional object contains a non-null value, get the non-null value, or perform some action if the Optional object contains a non-null value. By using the Optional class in your Java programs, you can write more reliable and maintainable code that is less prone to errors and easier to test.