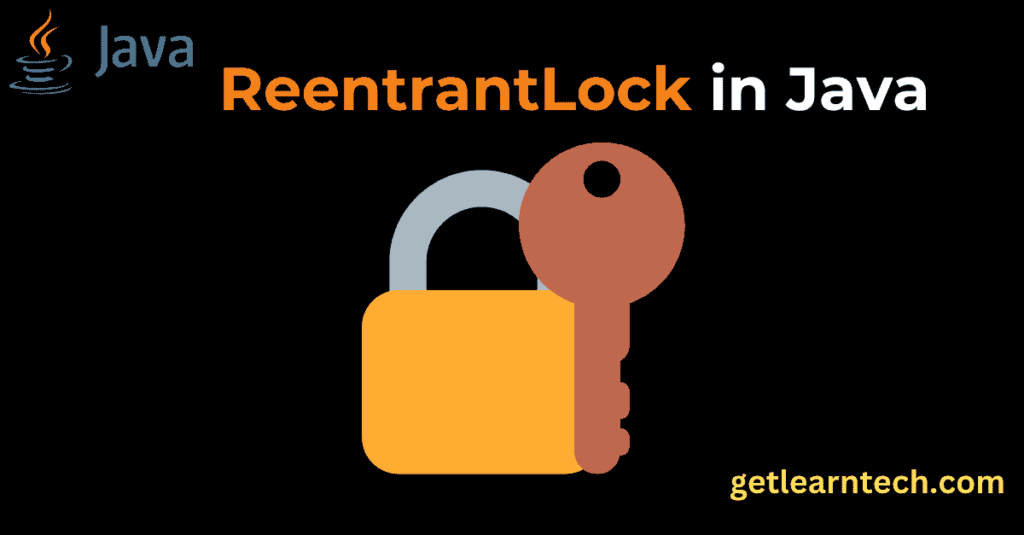
Table of Contents
Introduction to ReentrantLock in Java
Java provides several mechanisms for thread synchronization, and ReentrantLock
is one of the most powerful among them. ReentrantLock
, introduced in Java 5, is part of the java.util.concurrent.locks
package and offers more flexibility and control over thread synchronization than the traditional synchronized
keyword.
What is ReentrantLock?
A ReentrantLock
is a type of lock that allows the thread that holds the lock to re-acquire it without being blocked. It’s called “reentrant” because it allows the thread to enter the lock multiple times without causing a deadlock. This capability is particularly useful in recursive algorithms or when multiple methods in a thread need to lock the same resource.
Java ReentrantLock Example
Here’s a simple example of using ReentrantLock
:
import java.util.concurrent.locks.ReentrantLock;
public class ReentrantLockExample {
private final ReentrantLock lock = new ReentrantLock();
private int counter = 0;
public void increment() {
lock.lock();
try {
counter++;
} finally {
lock.unlock();
}
}
public int getCounter() {
return counter;
}
public static void main(String[] args) {
ReentrantLockExample example = new ReentrantLockExample();
// Creating threads to increment the counter
Thread t1 = new Thread(example::increment);
Thread t2 = new Thread(example::increment);
t1.start();
t2.start();
try {
t1.join();
t2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Counter: " + example.getCounter()); // Output should be 2
}
}
ReentrantLock vs Synchronized
Synchronized
The synchronized
keyword is a traditional way to achieve mutual exclusion in Java. It can be used to lock methods or code blocks, and the lock is automatically released when the method or block finishes execution.
ReentrantLock
ReentrantLock
provides all the features of synchronized
but with additional capabilities:
- TryLock: Attempt to acquire the lock without blocking.
- Lock Interruptibly: Acquire the lock unless the thread is interrupted.
- Fairness:
ReentrantLock
can be configured to enforce a first-come-first-served queue for acquiring the lock.
ReentrantLock vs Synchronized Performance
In terms of performance, ReentrantLock
can be more efficient in scenarios requiring high concurrency. The tryLock()
and lockInterruptibly()
methods offer more control over lock acquisition, potentially reducing contention and improving performance.
However, for simple scenarios, the overhead of managing ReentrantLock
manually (such as always ensuring unlock()
is called) may outweigh its benefits, making synchronized
more straightforward and less error-prone.
Java Lock vs ReentrantLock
ReentrantLock
is a specific implementation of the more general Lock
interface in Java, which provides more advanced thread synchronization features compared to the intrinsic lock that is implicit with the synchronized
keyword.
- Locks Interface: The
Lock
interface defines a locking mechanism where locks can be acquired and released in a more flexible way than intrinsic locks. For example, you can try to acquire a lock without blocking, specify a timeout for acquiring a lock, or acquire a lock that can be interrupted. - ReentrantLock: It is one of the most commonly used implementations of the
Lock
interface and is reentrant, meaning the same thread can acquire the lock multiple times without causing a deadlock.
ReentrantLock in Java 8 Example
Java 8 introduced several concurrency improvements, including enhancements to ReentrantLock
. Here’s an example demonstrating its usage with lambda expressions:
import java.util.concurrent.locks.ReentrantLock;
public class ReentrantLockJava8Example {
private final ReentrantLock lock = new ReentrantLock();
private int count = 0;
public void increment() {
lock.lock();
try {
count++;
} finally {
lock.unlock();
}
}
public int getCount() {
return count;
}
public static void main(String[] args) {
ReentrantLockJava8Example example = new ReentrantLockJava8Example();
Runnable task = example::increment;
Thread t1 = new Thread(task);
Thread t2 = new Thread(task);
t1.start();
t2.start();
try {
t1.join();
t2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Count: " + example.getCount()); // Output should be 2
}
}
ReentrantLock in Java 11
In Java 11, ReentrantLock
continues to offer the features introduced in earlier versions, with improvements and optimizations under the hood. While the API remains consistent, the internals have been optimized for better performance, especially in high-concurrency environments.
Intrinsic Lock vs ReentrantLock in Java
Intrinsic Lock:
- This is the lock that is implicitly acquired when a thread enters a synchronized block or method.
- It’s simple to use but lacks advanced features like fairness, timed lock attempts, or the ability to interrupt a thread waiting to acquire the lock.
ReentrantLock:
- Offers more flexibility, including the ability to try locking without blocking, interrupting a lock attempt, and ensuring fairness in lock acquisition.
- Requires manual lock and unlock, which means there is more responsibility to ensure the lock is released, but it also provides more control.
Heap vs Stack in Java
When discussing Java memory management, it’s crucial to distinguish between the heap and the stack:
- Heap: The heap is used for dynamic memory allocation. Objects are stored in the heap, and it is shared among all threads. If you want to know how heap data structure can be implemented in Java, then you can checkout.
- Stack: The stack is used for static memory allocation and local variables. Each thread has its own stack.
ReentrantLock
operates in the heap since it’s an object. Understanding heap and stack memory management is essential for efficiently managing resources in multithreaded applications.
FAQs
1. When should I use ReentrantLock
over synchronized
?
- Use
ReentrantLock
when you need more advanced features like fair locking, timed locking, or interruptible locking. For simpler use cases,synchronized
might be more appropriate.
2. Is ReentrantLock
faster than synchronized
?
- In high-concurrency situations,
ReentrantLock
can offer better performance due to its flexibility and advanced features. However, for simple locking, the difference may not be significant.
3. What is a fair lock in ReentrantLock
?
- A fair lock ensures that the longest-waiting thread acquires the lock next, which can prevent starvation but may lead to reduced throughput.
4. Can ReentrantLock
be used for reentrant functions?
- Yes,
ReentrantLock
allows the same thread to acquire the lock multiple times, making it suitable for recursive functions.
5. How does ReentrantLock
handle deadlocks?
ReentrantLock
does not inherently prevent deadlocks, but its features (liketryLock()
with a timeout) can help avoid them by allowing threads to back off.
Conclusion
Understanding ReentrantLock
in Java and its applications is vital for writing efficient, thread-safe Java code. Whether you need to manage complex concurrency patterns or simply need more control over locking mechanisms, ReentrantLock
provides a robust alternative to the synchronized
keyword. By comparing it with other synchronization techniques and understanding its role in Java’s concurrency framework, you can make informed decisions about when and how to use it in your projects.
I cherished as much as you’ll obtain carried out proper here. The cartoon is tasteful, your authored subject matter stylish. nevertheless, you command get bought an shakiness over that you would like be delivering the following. in poor health surely come more until now again as exactly the same just about a lot steadily inside of case you defend this hike.