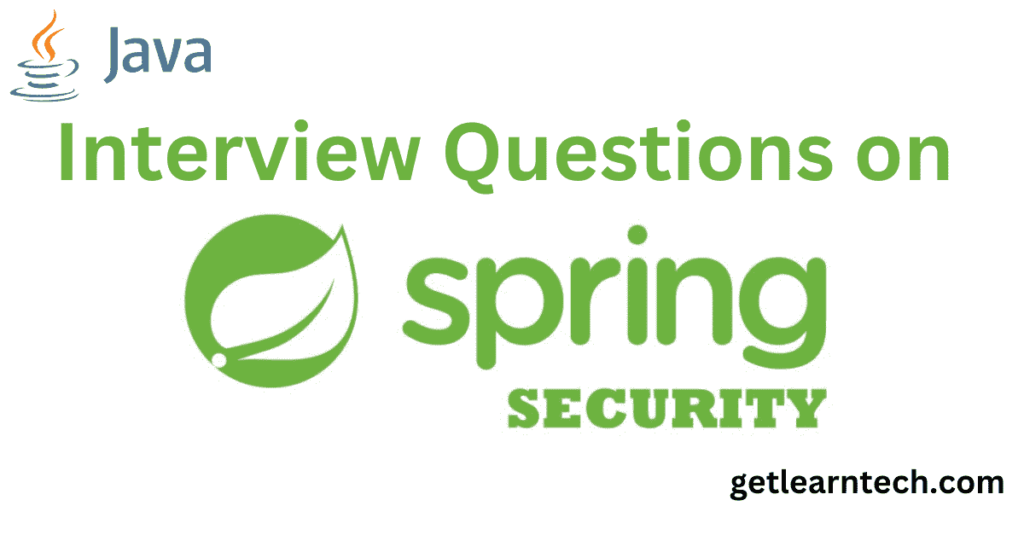
Table of Contents
Spring Security is an essential component for securing Spring-based applications and having a deep understanding of it is crucial for success in job interviews. Below, you’ll find detailed questions and answers tailored to different experience levels—3 years, 5 years, and 10 years. These questions cover fundamental to advanced topics in Spring Security.
Spring Security Interview Questions and Answers for 3 Years of Experience
For candidates with around 3 years of experience, the focus is typically on fundamental concepts and practical implementation.
1. What is Spring Security?
Answer:
Spring Security is a powerful and customizable authentication and access control framework for securing Spring-based Java applications. It provides comprehensive security services for Java EE-based enterprise software applications. The primary functions of Spring Security are to handle authentication (identity verification) and authorization (access control).
2. How does Spring Security handle authentication?
Answer:
Spring Security handles authentication by using the AuthenticationManager
interface, which processes authentication requests through a chain of AuthenticationProvider
instances. The most commonly used implementation of AuthenticationManager
is ProviderManager
. It uses UserDetailsService
to load user details from a data source (like a database) to verify credentials.
3. What are the different ways to configure Spring Security in a Spring application?
Answer:
Spring Security can be configured in three primary ways:
- Annotation-based Configuration: Using annotations like
@EnableWebSecurity
and@Configuration
to configure security in a Java class. - XML Configuration: Using an XML file to define security configurations, which was more common in older Spring applications.
- Java-based Configuration: Writing security configurations directly in a Java class, which is the modern and preferred approach.
4. What is a Security Filter Chain in Spring Security?
Answer:
The Security Filter Chain in Spring Security is a series of filters that the incoming HTTP requests pass through before reaching the application. Each filter performs a specific security function (e.g., authentication, authorization). The filters are executed in a specific order, starting from SecurityContextPersistenceFilter
to ExceptionTranslationFilter
.
5. How do you implement role-based access control (RBAC) in Spring Security?
Answer:
Role-based access control in Spring Security can be implemented using annotations like @Secured
, @PreAuthorize
, and @RolesAllowed
. These annotations are placed on controller methods or service methods to restrict access based on user roles.
6. How does Spring Security handle CSRF (Cross-Site Request Forgery) attacks?
Answer:
Spring Security mitigates CSRF attacks by generating a unique token for each session and attaching it to each HTTP request that changes state (e.g., POST, PUT, DELETE). This token is validated on the server side before processing the request, ensuring that the request originated from an authenticated source.
7. What is UserDetails
in Spring Security?
Answer:
The UserDetails
interface represents a core user information model in Spring Security. It contains methods to retrieve the username, password, and authorities of the user. Custom implementations of UserDetails
are often used to integrate Spring Security with custom user data models.
8. How do you secure REST APIs using Spring Security?
Answer:
To secure REST APIs in Spring Security, you typically use token-based authentication mechanisms like JWT (JSON Web Tokens). Basic authentication or OAuth2 can also be employed. Security is enforced at the controller level using @PreAuthorize
annotations or via the security filter chain.
9. What is the purpose of SecurityContextHolder
in Spring Security?
Answer:SecurityContextHolder
is a central component in Spring Security that stores the security context, including details about the currently authenticated user. This information is accessible throughout the application and can be retrieved using SecurityContextHolder.getContext().getAuthentication()
.
10. How do you configure password encoding in Spring Security?
Answer:
Password encoding in Spring Security is configured using PasswordEncoder
. The most commonly used encoder is BCryptPasswordEncoder
, which hashes the password using the BCrypt hashing algorithm. The encoder is typically set in the security configuration class.
Spring Security Interview Questions and Answers for 5 Years of Experience
With 5 years of experience, candidates are expected to have a deeper understanding of Spring Security, including advanced features and customization.
1. What is the role of GrantedAuthority
in Spring Security?
Answer:GrantedAuthority
represents an authority granted to the user, such as a role or a privilege. It is used in conjunction with Authentication
objects to define what actions a user is authorized to perform. Each GrantedAuthority
is usually a string representing a role like “ROLE_USER” or “ROLE_ADMIN”.
2. How do you customize authentication in Spring Security?
Answer:
Custom authentication can be implemented by creating a custom AuthenticationProvider
and overriding the authenticate
method. Additionally, a custom UserDetailsService
can be used to load user-specific data from a database or an external system.
3. What is the purpose of SecurityContextHolder
in Spring Security?
Answer:SecurityContextHolder
holds the security context of the current application context. It stores the details of the authenticated user, such as credentials and granted authorities. The security context can be accessed globally using SecurityContextHolder.getContext()
.
4. How do you implement JWT authentication in Spring Security?
Answer:
JWT authentication involves generating a JSON Web Token after successful authentication, and then validating this token on subsequent requests. The token is usually passed in the Authorization
header of HTTP requests. Custom filters are created to intercept requests and validate the JWT before allowing access to secured resources.
5. How do you handle method-level security in Spring Security?
Answer:
Method-level security is handled using annotations like @PreAuthorize
, @PostAuthorize
, @Secured
, and @RolesAllowed
. These annotations are placed above methods in services or controllers to enforce security constraints based on user roles or permissions.
6. What is OAuth2 and how does Spring Security support it?
Answer:
OAuth2 is an authorization framework that allows third-party services to exchange credentials and access resources without exposing user credentials. Spring Security provides comprehensive support for OAuth2, enabling the implementation of both OAuth2 resource servers and authorization servers.
7. How do you integrate Spring Security with LDAP?
Answer:
Spring Security can be integrated with LDAP for authentication by configuring an LdapAuthenticationProvider
. The UserDetailsService
is customized to query LDAP directories for user information. Spring Security also supports LDAP group-based access control.
8. What is SecurityContextPersistenceFilter
and its role in Spring Security?
Answer:SecurityContextPersistenceFilter
is responsible for storing the SecurityContext
between requests in a session or other persistence mechanism. This ensures that security context information is available throughout the session.
9. How do you configure remember-me authentication in Spring Security?
Answer:
Remember-me authentication is configured using the RememberMeServices
interface or PersistentTokenBasedRememberMeServices
. This feature allows users to stay authenticated across sessions by storing a token in the client (usually as a cookie) that is validated on subsequent requests.
10. How do you secure a Spring Boot application using Spring Security?
Answer:
Securing a Spring Boot application involves including the Spring Security starter dependency and configuring security rules using WebSecurityConfigurerAdapter
. By default, all endpoints are secured, and custom security configurations are made to specify which endpoints require authentication or are publicly accessible.
Spring Security Interview Questions and Answers for 10 Years of Experience
Professionals with 10 years of experience are expected to answer questions on architectural considerations, performance optimizations, and advanced integrations.
1. How do you architect a secure microservices-based application using Spring Security?
Answer:
Securing microservices involves using techniques such as OAuth2 for token-based authentication, API gateways for centralized security, and service-to-service authentication using mutual TLS. Each microservice can be secured independently, and user identity is propagated using JWT tokens across microservices.
2. What are some common security vulnerabilities in Spring applications, and how do you mitigate them?
Answer:
Common security vulnerabilities include:
- SQL Injection: Mitigated by using parameterized queries or ORM tools like Hibernate.
- Cross-Site Scripting (XSS): Prevented by validating and escaping user inputs.
- Cross-Site Request Forgery (CSRF): Mitigated using CSRF tokens.
3. How do you optimize the performance of a Spring Security-enabled application?
Answer:
Performance optimization strategies include:
- Caching authentication data to reduce database calls.
- Optimizing the filter chain to minimize the number of filters applied to each request.
- Using stateless sessions where possible to reduce server overhead.
4. How do you integrate Spring Security with SSO (Single Sign-On) solutions?
Answer:
Spring Security can be integrated with SSO solutions like SAML, OAuth2, or OpenID Connect. This involves configuring the application as an OAuth2 client or SAML service provider, enabling centralized authentication across multiple applications.
5
. How do you secure RESTful APIs in a distributed system using Spring Security?
Answer:
RESTful APIs in distributed systems are secured using strategies such as:
- Token-based authentication (e.g., JWT) for stateless security.
- Mutual TLS for secure communication between services.
- API gateways to centralize security and manage API access.
6. How do you manage security in a cloud-native Spring application?
Answer:
Managing security in cloud-native applications involves:
- Using cloud provider security tools like AWS IAM, Azure AD, or Google Cloud Identity.
- Integrating with Kubernetes RBAC for managing access controls in containerized environments.
- Encrypting sensitive data and using secrets management tools.
7. What is the role of AccessDecisionManager
in Spring Security?
Answer:AccessDecisionManager
is responsible for making final authorization decisions in Spring Security. It evaluates the Authentication
object against ConfigAttribute
objects defined for secured resources. The AccessDecisionManager
delegates to a list of AccessDecisionVoter
s, which vote on whether access should be granted.
8. How do you handle security in a reactive Spring application?
Answer:
In reactive Spring applications, security is managed using WebFluxSecurity
configurations. Reactive security involves non-blocking operations, and security contexts are propagated using reactive data structures like Mono
and Flux
.
9. How do you implement custom security auditing in Spring Security?
Answer:
Custom security auditing can be implemented by creating event listeners for Spring Security events like AuthenticationSuccessEvent
or AuthorizationFailureEvent
. Auditing can be enhanced by logging these events or storing them in a database for analysis.
10. What are the best practices for securing a Spring-based enterprise application?
Answer:
Best practices include:
- Using the principle of least privilege for user roles and permissions.
- Encrypting sensitive data both in transit and at rest.
- Regularly updating dependencies to mitigate known vulnerabilities.
- Implementing comprehensive logging and monitoring for security events.
Conclusion
Spring Security is a vital framework for securing enterprise applications built with Spring. By preparing for the Spring Security Interview questions outlined above, you can build a strong foundation in Spring Security, regardless of your experience level. Whether you are a beginner or a seasoned expert, understanding these concepts will help you excel in interviews and secure your next role.
This comprehensive guide covers essential Spring Security interview questions and answers for 3, 5, and 10 years of experience, ensuring you’re well-prepared for any level of questioning.
If you are preparing for microservices interviews then checkout here.
If you are preparing for Java interviews you can checkout here.
I have not checked in here for some time because I thought it was getting boring, but the last several posts are great quality so I guess I?¦ll add you back to my everyday bloglist. You deserve it my friend 🙂