When preparing for a Java developer interview, it is crucial to be well-versed with various aspects of Java Strings. This article covers common Java String interview questions and their answers to help you succeed in your interview.
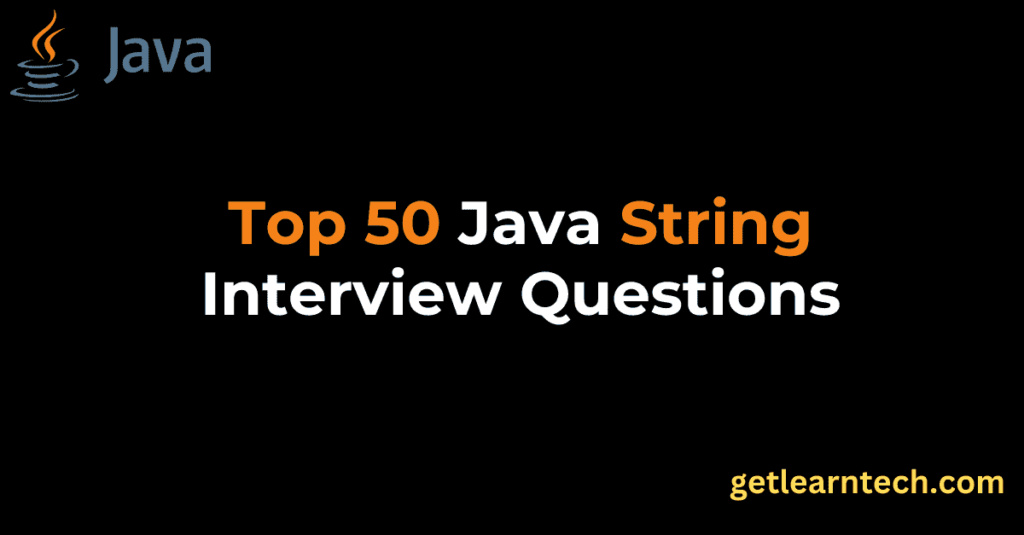
Table of Contents
Java String Interview Questions and answers
1. What are Strings and What are the different ways to create string objects in Java?
- In Java, a string is an object that represents a sequence of characters or char values. The
java.lang.String
class is used to create a Java string object. - Strings are sequences of characters and are stored in 16 bits using UTF 16-bit encoding. Each character is stored as an array of characters in Java.
- Strings are immutable in Java, meaning their values cannot be changed after creation. This immutability allows strings to be shared and optimized for memory efficiency.
To create String objects in Java, there are two main methods: using string literals and using the new
keyword. Here are examples of both methods based on the provided sources:
Creating Strings Using String Literals:
- String literals involve assigning a string value wrapped in double quotes to a String type variable. This method creates strings that are stored in the string constant pool in memory.
- Example:
String message = "Hello there";
Creating Strings Using the new
Keyword:
- When using the
new
keyword, a new String object is created in the heap memory. This method does not store the string in the string pool but directly in the heap memory. - Example:
String name = new String("Java String");
Key Points:
- String literals create strings that are stored in the string constant pool, allowing for reuse of existing strings with the same value.
- Using the
new
keyword creates a new object in the heap memory, even if the string value already exists in the pool.
In Java, strings are objects of the String
class, and understanding the differences between creating strings with string literals and the new
keyword is essential for efficient memory management and string manipulation in Java programs.
2. What is the String pool in Java?
String pool is a special storage space in the Java heap memory where string literals are stored. This pool helps in saving memory and improving performance by reusing instances of strings that have the same value.
When a string literal is created in Java, the JVM checks the String pool to see if an identical string already exists. If it does, the reference to the existing string is returned instead of creating a new one.
String str1 = "hello";
String str2 = "hello";
System.out.println(str1 == str2); // true
Here, str1
and str2
point to the same object in the String pool.
The String pool helps in reducing memory usage by storing only one copy of each literal string, thus avoiding duplicate strings in the memory.
3. What is the difference between ==
and equals()
method in Java?
==
Operator: This compares the references (memory addresses) of the two objects, not their actual content.equals()
Method: This method compares the actual content of the objects. For strings, it checks if the characters in the strings are the same.
String s1 = new String("Hello");
String s2 = new String("Hello");
System.out.println(s1 == s2); // false
System.out.println(s1.equals(s2)); // true
4. How does the intern()
method work in Java?
The intern()
method in Java returns a canonical representation for the string object. When a string’s intern()
method is invoked, Java checks the string pool to see if an identical string already exists. If it does, the reference from the pool is returned; otherwise, the string is added to the pool and its reference is returned.
String s1 = new String("Hello").intern();
String s2 = "Hello";
System.out.println(s1 == s2); // true
5. Explain immutability in Java Strings.
Strings in Java are immutable, meaning once a String
object is created, its value cannot be changed. Any operation that modifies a string will result in a new String
object being created.
Why are Strings immutable in Java or advantages of Strings immutability?
- Security: Prevents inadvertent or malicious changes to string data.
- Synchronization: Immutable objects are inherently thread-safe, eliminating synchronization issues.
- Caching and Performance: Allows the JVM to cache string literals and reuse them, reducing memory overhead.
String s1 = "Hello";
s1.concat(" World");
System.out.println(s1); // "Hello" -- You can't change String object, once it is
created
String s2 = s1.concat(" World");
System.out.println(s2); // "Hello World"
6. What are the differences between String
, StringBuilder
, and StringBuffer
?
String
: Immutable, any modification creates a new string.StringBuilder
: Mutable, not thread-safe, faster performance. It is faster thanStringBuffer
because it is not synchronized.StringBuffer
: Mutable, thread-safe, slightly slower then StringBuilder due to synchronization and thread safety.
7. What is the StringJoiner
class used for?
StringJoiner
is a class in Java that constructs a sequence of characters separated by a delimiter and optionally starting with a supplied prefix and ending with a supplied suffix.
StringJoiner joiner = new StringJoiner(", ", "[", "]");
# Here delimiter is ",", Prefix is "[", Suffix is "]"
joiner.add("apple").add("banana").add("orange");
System.out.println(joiner); // [apple, banana, orange]
8. How can you convert a String
to a char
array?
You can use the toCharArray()
method to convert a string to a character array.
String str = "Hello";
char[] charArray = str.toCharArray();
for (char c : charArray) {
System.out.print(c + " "); // H e l l o
}
9. Explain the substring()
method in Java. What are the common pitfalls of using the substring()
method?
The substring()
method extracts a part of the string starting from a specified index to the end or up to another specified index.
String str = "Hello, World!";
String subStr1 = str.substring(7); // "World!"
String subStr2 = str.substring(7, 12); // "World"
Common pitfalls include:
- OutOfBoundsException: If the indices are out of range.
- Memory Leaks: Prior to Java 7,
substring()
would create memory leaks by retaining references to the original string’s character array.
10. What is the significance of the charAt()
method?
The charAt()
method returns the character at a specified index in a string
String str = "Hello";
char ch = str.charAt(1); // 'e'
11. Explain the use of StringTokenizer
class.
StringTokenizer
is a legacy class used to break a string into tokens. It is not recommended for new code because split()
or Scanner
is preferred due to more flexibility.
String str = "apple banana orange";
StringTokenizer tokenizer = new StringTokenizer(str);
while (tokenizer.hasMoreTokens()) {
System.out.println(tokenizer.nextToken());
}
12. What is the concat()
method in Java?
The concat()
method is used to concatenate two strings.
String str1 = "Hello";
String str2 = "World";
String str3 = str1.concat(str2); // "HelloWorld"
13. How do you convert a String to an integer in Java?
Use the Integer.parseInt()
method to convert a string to an integer.
String str = "123";
int num = Integer.parseInt(str); // 123
14. What is contains method does in Strings?
The contains()
method in Java is used to check if a specific sequence of characters is present within a given string. It returns a boolean value, true
if the sequence is found in the string, and false
if it is not found.
public class StringContainsExample {
public static void main(String[] args) {
String fruit = "apple";
// Checking if the string contains a specific substring
boolean containsApple = fruit.contains("app");
System.out.println("Contains 'app'? " + containsApple); // Output: Contains 'app'? true
boolean containsBanana = fruit.contains("banana");
System.out.println("Contains 'banana'? " + containsBanana); // Output: Contains 'banana'? false
}
}
15. How do you remove leading and trailing whitespace from a string?
Use the trim()
method to remove leading and trailing whitespace.
String str = " Hello World ";
String trimmedStr = str.trim(); // "Hello World"
16. How do you check if a string is empty or null?
Using isEmpty()
: Checks if the string is empty.
String str = "";
boolean isEmpty = str.isEmpty(); // true
Using == null
: Checks if the string is null.
String str = null;
boolean isNull = (str == null); // true
Advanced Java String Interview Questions and Answers for Experienced Developers
As Java continues to evolve, new features and improvements are added to the language, including enhancements to string handling. If you have around five years of experience, being familiar with the latest features and updates in Java can give you an edge in interviews. Here are some advanced Java String interview questions and answers, including those from the latest Java versions.
17. What new String methods were introduced in Java 11?
Java 11 introduced several new methods to the String
class to enhance its functionality:
isBlank()
: Checks if the string is empty or contains only white space.lines()
: Returns a stream of lines extracted from the string, separated by line terminators.strip()
,stripLeading()
,stripTrailing()
: Removes white space from both ends, the beginning and the end of the string respectively.repeat(int count)
: Returns a string whose value is the concatenation of the given string repeatedcount
times.
String str = " Hello ";
System.out.println(str.isBlank()); // false
System.out.println(str.strip()); // "Hello"
System.out.println("Java\nis\nfun".lines().collect(Collectors.toList())); // [Java, is, fun]
System.out.println("abc".repeat(3)); // "abcabcabc"
18. How can you remove leading and trailing whitespace from a string using methods introduced in Java 11?
Java 11 introduced the strip()
, stripLeading()
, and stripTrailing()
methods for removing whitespace.
String str = " Hello World ";
System.out.println(str.strip()); // "Hello World"
System.out.println(str.stripLeading()); // "Hello World "
System.out.println(str.stripTrailing()); // " Hello World"
19. What are the performance implications of using String
concatenation in a loop, and how can you mitigate them?
Using String
concatenation in a loop can lead to performance issues because each concatenation creates a new String
object. This can be mitigated by using StringBuilder
(or StringBuffer
for thread safety), which allows for more efficient string manipulation.
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append(i);
}
String result = sb.toString();
System.out.println(result);
20. How do you format a string to display a date in a specific pattern?
You can use String.format()
along with SimpleDateFormat
to format a date into a specific pattern.
import java.text.SimpleDateFormat;
import java.util.Date;
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String formattedDate = String.format("Date: %s", sdf.format(date));
System.out.println(formattedDate); // "Date: 2024-05-22"
21. How do you compare two strings lexicographically?
You can use the compareTo()
method to compare two strings lexicographically.
String str1 = "apple";
String str2 = "banana";
int comparison = str1.compareTo(str2);
System.out.println(comparison); // negative value because "apple" is lexicographically less than "banana"
22. How does the String
class’s indent()
method work in Java 12?
The indent()
method, introduced in Java 12, adjusts the indentation of each line in the string. Positive values add spaces to the beginning of each line, and negative values remove spaces.
String str = "Hello\nWorld";
System.out.println(str.indent(4));
// Output:
// " Hello
// World"
System.out.println(str.indent(-2));
// Output:
// "Hello
// World"
23. Explain the transform()
method introduced in Java 12.
The transform()
method applies a function to the string and returns the result. It allows chaining of string manipulations in a more readable manner.
String result = "hello"
.transform(s -> s + " world")
.transform(String::toUpperCase);
System.out.println(result); // "HELLO WORLD"
24. What improvements were made to the String
class in Java 13?
Java 13 introduced text blocks, which simplify the inclusion of multi-line strings in Java code by using triple quotes ("""
). Text blocks preserve the format of the string content as written in the source code. It helps in writing strings that span multiple lines and improves readability and maintainability of code that deals with multi-line strings.
String textBlock = """
{
"name": "John",
"age": 30,
"city": "New York"
}
""";
System.out.println(textBlock);
25. Describe the formatted()
method introduced in Java 15.
The String.format()
method allows you to create formatted strings using format specifiers.
String formattedString = String.format("Name: %s, Age: %d", "Alice", 30);
// "Name: Alice, Age: 30"
The formatted()
provides a more concise way to format strings. It is a syntactic sugar over String.format()
, making the code more readable and reducing verbosity.
String str = "Hello, %s!".formatted("World");
System.out.println(str); // "Hello, World!"
26. How can you join strings using String.join()
method?
The String.join()
method joins multiple strings with a specified delimiter.
String joined = String.join(", ", "apple", "banana", "cherry");
System.out.println(joined); // "apple, banana, cherry"
27. How to use the chars()
and codePoints()
methods?
The chars()
method returns an IntStream
of char values from the string, while the codePoints()
method returns an IntStream
of Unicode code points.
String str = "abc";
str.chars().forEach(ch -> System.out.print((char) ch + " ")); // a b c
System.out.println();
str.codePoints().forEach(cp -> System.out.print((char) cp + " ")); // a b c
28. What is the difference between substring()
and subSequence()
?
The substring()
and subSequence()
methods in Java are used to extract a portion of a string, but they have some differences:
substring()
:
- The
substring()
method returns a new string that is a substring of the original string. - It extracts a contiguous sequence of characters from the original string.
- The extracted substring includes the character at the start index and up to, but not including, the character at the end index.
- Example:
"Hello".substring(1, 4)
returns “ell”.
subSequence()
:
- The
subSequence()
method returns a newCharSequence
that is a subsequence of the original string. - It extracts a sequence of characters from the original string, but the extracted characters do not need to be contiguous.
- The
CharSequence
returned bysubSequence()
is read-only, meaning you cannot change the characters within it without creating a new instance. - Example:
"Hello".subSequence(1, 4)
returns aCharSequence
containing “ell”.
Key differences::
- Contiguity: Substrings are contiguous, while subsequences can be non-contiguous.
- Return type:
substring()
returns aString
, whilesubSequence()
returns aCharSequence
. - Mutability: The
CharSequence
returned bysubSequence()
is read-only, while theString
returned bysubstring()
can be modified.
In summary, substring()
extracts a contiguous portion of a string, while subSequence()
extracts a sequence of characters that may or may not be contiguous. The main practical difference is that substring()
returns a modifiable String
, while subSequence()
returns a read-only CharSequence
.
String str = "Hello, World!";
String substr = str.substring(7); // "World!"
CharSequence subseq = str.subSequence(7, 12); // "World"
29. Explain the matches()
method in the String
class.
The matches()
method checks if the string matches a given regular expression.
String str = "abc123";
boolean isMatch = str.matches("\\w+\\d+"); // true
30. How can you convert a String
to an IntStream
of code points in Java 9?
In Java 9, you can convert a String
to an IntStream
of code points using the codePoints()
method.
String str = "abc";
IntStream codePoints = str.codePoints();
codePoints.forEach(System.out::println); // 97 98 99 (ASCII values of 'a', 'b', 'c')
31. How do you convert a String
to a stream of characters using chars()
?
The chars()
method returns an IntStream
of the char values in the String
. You can then use this stream to process or manipulate the characters.
String str = "hello";
str.chars().forEach(c -> System.out.print((char) c + " ")); // h e l l o
32. How do you join/append multiple strings in Java other then using StringBuilder and StringBuffer?
Use the String.join()
method or StringJoiner
class.
Using String.join()
String joinedString = String.join(", ", "one", "two", "three");
System.out.println(joinedString);
// "one, two, three"
Using StringJoiner
StringJoiner joiner = new StringJoiner(", ");
joiner.add("one");
joiner.add("two");
joiner.add("three");
String joinedString = joiner.toString();
// "one, two, three"
String programming interview questions in Java for freshers and experienced
33. How can you check if a string contains only digits?
String str = "12345";
boolean isNumeric = str.matches("\\d+"); // true
34. How do you check if a string starts/ends with a specific substring?
To check if a string starts with a substring:
String text = "Hello, world!";
boolean startsWithHello = text.startsWith("Hello");
To check if a string ends with a substring:
String filename = "example.txt";
boolean endsWithTxt = filename.endsWith(".txt");
35. How can you reverse a String in Java?
You can reverse a string using the StringBuilder
or StringBuffer
class, which provide a reverse()
method.
String str = "Hello";
String reversed = new StringBuilder(str).reverse().toString();
System.out.println(reversed); // "olleH"
36. How can you check if two strings are anagrams?
To check if two strings are anagrams, you can sort both strings and compare them.
public boolean areAnagrams(String s1, String s2) {
char[] arr1 = s1.toCharArray();
char[] arr2 = s2.toCharArray();
Arrays.sort(arr1);
Arrays.sort(arr2);
return Arrays.equals(arr1, arr2);
}
37. How do you find the first non-repeated character in a string?
public char firstNonRepeatedChar(String str) {
Map<Character, Integer> charCount = new LinkedHashMap<>();
for (char ch : str.toCharArray()) {
charCount.put(ch, charCount.getOrDefault(ch, 0) + 1);
}
for (Map.Entry<Character, Integer> entry : charCount.entrySet()) {
if (entry.getValue() == 1) {
return entry.getKey();
}
}
return '\0'; // Return a null character if no non-repeated character found
}
38. How can you check if a string is a palindrome?
public boolean isPalindrome(String str) {
int left = 0, right = str.length() - 1;
while (left < right) {
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
39. How do you remove duplicate characters from a string?
You can use a LinkedHashSet
to maintain the order of characters and remove duplicates.
public String removeDuplicates(String str) {
Set<Character> seen = new LinkedHashSet<>();
for (char ch : str.toCharArray()) {
seen.add(ch);
}
StringBuilder sb = new StringBuilder();
for (char ch : seen) {
sb.append(ch);
}
return sb.toString();
}
40. How can you count the number of words in a string?
You can split the string by spaces or use a regular expression to count the words.
public int countWords(String str) {
if (str == null || str.isEmpty()) {
return 0;
}
String[] words = str.trim().split("\\s+");
return words.length;
}
41. How do you check if a string contains only alphabets?
You can use a regular expression to check if a string contains only alphabetic characters.
public boolean isAlphabetic(String str) {
return str != null && str.matches("[a-zA-Z]+");
}
42. How do you replace all occurrences of a substring in a string?
You can use the replaceAll()
method to replace all occurrences of a substring.
String str = "hello world, hello universe";
String replacedStr = str.replaceAll("hello", "hi");
System.out.println(replacedStr); // "hi world, hi universe"
43. How do you convert a String
to an int
in Java?
You can use the Integer.parseInt()
method to convert a String
to an int
.
String str = "1234";
int num = Integer.parseInt(str);
System.out.println(num); // 1234
44. How can you compare two strings lexicographically ignoring case differences?
You can use the compareToIgnoreCase()
method to compare two strings lexicographically, ignoring case differences.
String str1 = "Hello";
String str2 = "hello";
int comparison = str1.compareToIgnoreCase(str2);
System.out.println(comparison); // 0, because they are equal ignoring case
45. How do you split a string by a specific character?
You can use the split()
method to split a string by a specific character.
String str = "apple,banana,orange";
String[] fruits = str.split(",");
for (String fruit : fruits) {
System.out.println(fruit);
}
// Output:
// apple
// banana
// orange
46. How can you convert a String
to a char
array and back?
You can use the toCharArray()
method to convert a String
to a char
array and the String
constructor to convert a char
array back to a String
.
String str = "hello";
char[] charArray = str.toCharArray();
String newStr = new String(charArray);
System.out.println(newStr); // "hello"
47. How do you find the longest palindromic substring in a string?
You can use a dynamic programming approach or expand around the center to find the longest palindromic substring.
public String longestPalindrome(String s) {
if (s == null || s.length() < 1) return "";
int start = 0, end = 0;
for (int i = 0; i < s.length(); i++) {
int len1 = expandAroundCenter(s, i, i);
int len2 = expandAroundCenter(s, i, i + 1);
int len = Math.max(len1, len2);
if (len > end - start) {
start = i - (len - 1) / 2;
end = i + len / 2;
}
}
return s.substring(start, end + 1);
}
private int expandAroundCenter(String s, int left, int right) {
while (left >= 0 && right < s.length() && s.charAt(left) == s.charAt(right)) {
left--;
right++;
}
return right - left - 1;
}
48. How do you find the length of the longest substring without repeating characters?
You can use a sliding window approach with a hash set to find the length of the longest substring without repeating characters.
public int lengthOfLongestSubstring(String s) {
Set<Character> set = new HashSet<>();
int maxLength = 0, left = 0, right = 0;
while (right < s.length()) {
if (!set.contains(s.charAt(right))) {
set.add(s.charAt(right));
right++;
maxLength = Math.max(maxLength, right - left);
} else {
set.remove(s.charAt(left));
left++;
}
}
return maxLength;
}
49. How do you reverse the words in a given sentence without reversing the individual words?
public String reverseWords(String sentence) {
if (sentence == null || sentence.isEmpty()) {
return sentence;
}
String[] words = sentence.split("\\s+");
Collections.reverse(Arrays.asList(words));
return String.join(" ", words);
}
// Example usage:
String sentence = "Hello World from Java";
String reversedSentence = reverseWords(sentence);
System.out.println(reversedSentence); // "Java from World Hello"
50. How do you check if a string can be formed by rotating another string?
public boolean isRotation(String s1, String s2) {
if (s1.length() != s2.length() || s1.length() == 0) {
return false;
}
String concatenated = s1 + s1;
return concatenated.contains(s2);
}
// Example usage:
String s1 = "waterbottle";
String s2 = "erbottlewat";
boolean isRotation = isRotation(s1, s2);
System.out.println(isRotation); // true
By being familiar with these advanced features and updates in the latest Java versions, you can showcase your deep knowledge of Java and impress your interviewers.
By preparing these Java string interview questions you will be well-equipped to handle Java string-related questions in your interview. Remember, practice is key to mastering these concepts and demonstrating your expertise effectively. Good luck!