Introduction
Python is one of the most sought-after programming languages, appreciated for its simplicity and extensive libraries. Whether you are a fresher or an experienced developer, preparing for a Python interview can be a rigorous task. This Python Interview Questions and Answers pdf includes 50 essential Python interview questions and answers, tailored for both freshers and experienced candidates to help you ace your next interview.
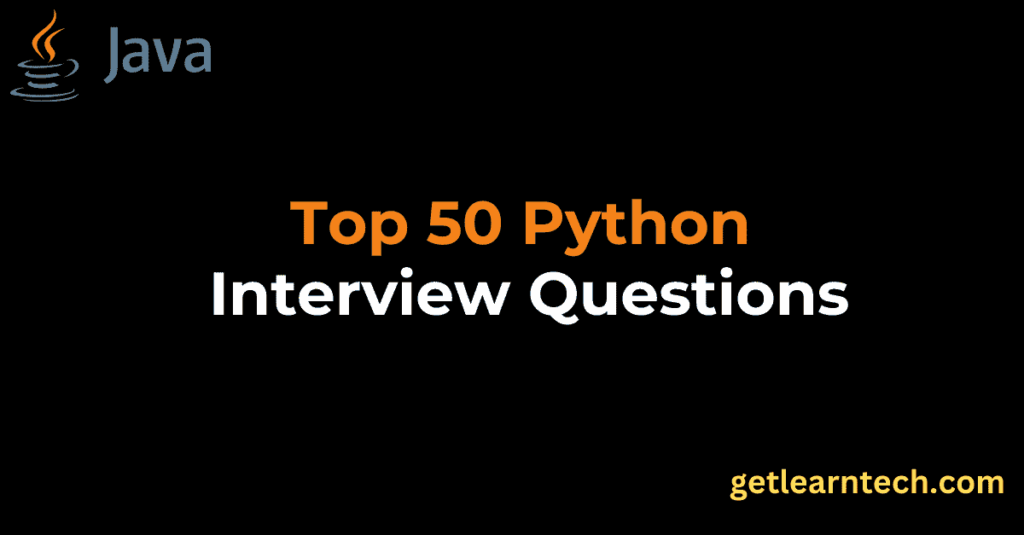
Table of Contents
You can download python interview questions and answers pdf here
Python Interview Questions and Answers for Freshers
1. What is Python?
Python is a high-level, interpreted programming language known for its readability and simplicity. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
2. Explain the basic data types in Python.
Python includes several built-in data types:
- Integers: Whole numbers, e.g.,
1, 2, 3
- Floats: Decimal numbers, e.g.,
1.0, 2.5
- Strings: Sequence of characters, e.g.,
"Hello, World!"
- Lists: Ordered, mutable collections, e.g.,
[1, 2, 3]
- Tuples: Ordered, immutable collections, e.g.,
(1, 2, 3)
- Dictionaries: Key-value pairs, e.g.,
{'a': 1, 'b': 2}
3. What are Python functions? How do you define them?
Functions in Python are reusable blocks of code that perform a specific task. They are defined using the def
keyword followed by the function name and parentheses ()
. Example:
def greet(name):
return f"Hello, {name}!"
4. Explain the concept of a Python virtual environment.
A virtual environment in Python is an isolated environment that allows you to manage dependencies for different projects separately. It ensures that each project can have its own dependencies, regardless of what dependencies every other project has.
5. What are Python modules and packages?
- Modules: A file containing Python code, which can define functions, classes, and variables. Example:
math
module. - Packages: A way of organizing related modules into a directory hierarchy. Example:
numpy
package.
6. How do you handle exceptions in Python?
Exceptions in Python are handled using try
, except
, else
, and finally
blocks. The try
block contains code that might throw an exception, while except
blocks handle specific exceptions.
7. What is PEP 8 and why is it important?
PEP 8 is the Python Enhancement Proposal that provides guidelines and best practices for writing Python code. Following PEP 8 helps maintain readability and consistency in the codebase.
8. How do you manage dependencies in a Python project?
Dependencies in a Python project are typically managed using pip
and a requirements.txt
file, where all required packages are listed.
9. What is a list comprehension and give an example?
List comprehensions provide a concise way to create lists. Example:
squares = [x**2 for x in range(10)]
10. Explain the difference between append()
and extend()
in lists.
append()
: Adds a single element to the end of a list.extend()
: Adds multiple elements to the end of a list.
11. What are decorators in Python?
Decorators are a way to modify or extend the behavior of functions or methods without changing their actual code. They are implemented as higher-order functions.
12. Explain the difference between shallow copy and deep copy.
- Shallow Copy: Creates a new object but inserts references into it to the objects found in the original.
- Deep Copy: Creates a new object and recursively adds copies of nested objects found in the original.
13. What is the purpose of self
in Python classes?
self
represents the instance of the class and is used to access variables and methods associated with the class.
14. How does Python handle memory management?
Python uses an automatic garbage collector to manage memory, primarily based on reference counting and a cyclic garbage collector to handle circular references.
15. What is the difference between __str__
and __repr__
methods?
__str__
: Used to define the “informal” or nicely printable string representation of an object.__repr__
: Used to define the “official” string representation of an object, ideally one that could be used to recreate the object.
16. Explain the usage of with
statement and context managers.
The with
statement is used to wrap the execution of a block of code. Context managers are used to manage resources such as file streams. The __enter__
and __exit__
methods define what happens before and after the block of code.
17. What are lambda functions in Python?
Lambda functions are small anonymous functions defined using the lambda
keyword. Example:
add = lambda x, y: x + y
18. What is the map()
function and how is it used?
The map()
function applies a given function to all items in an input list. Example:
numbers = [1, 2, 3, 4]
squares = list(map(lambda x: x**2, numbers))
19. Explain the difference between remove()
, pop()
, and del
in lists.
remove()
: Removes the first occurrence of a specified value.pop()
: Removes an element at a specified position and returns it.del
: Deletes an element at a specified position or the entire list.
20. How do you work with JSON data in Python?
Python provides the json
module to work with JSON data. You can use json.dumps()
to convert a Python object to a JSON string and json.loads()
to convert a JSON string to a Python object.
Python Interview Questions and Answers for Experienced
21. What is the Global Interpreter Lock (GIL) in Python?
The GIL is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecodes simultaneously in a multi-threaded program. This can be a bottleneck in CPU-bound and multi-threaded code.
22. Explain how Python’s garbage collection works.
Python’s garbage collection is primarily based on reference counting. An object is deleted when its reference count drops to zero. Additionally, Python has a cyclic garbage collector that can detect and clean up cyclic references.
23. What are Python generators?
Generators are a type of iterable, like lists or tuples. Unlike lists, they don’t allow indexing with arbitrary indices, but they can be iterated through with for
loops. They are created using functions and the yield
statement.
24. How do you use decorators in Python? Provide an example use case.
Decorators are used to modify the behavior of a function or method. Example use case:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
25. Explain the differences between Python 2 and Python 3.
Some key differences include:
- Print statement:
print "Hello"
in Python 2 vsprint("Hello")
in Python 3. - Integer division:
5/2
yields2
in Python 2 vs2.5
in Python 3. - Unicode: Strings are Unicode by default in Python 3.
26. What is the purpose of the pass
statement in Python?
The pass
statement is a null operation; it is used when a statement is syntactically required but you do not want any command or code to execute.
27. How can you optimize performance when working with large data sets in Python?
Strategies include using generators, leveraging efficient libraries like NumPy and pandas, minimizing memory usage, and using built-in functions and libraries.
28. Describe the role of the __init__.py
file in Python packages.
The __init__.py
file is used to mark directories on disk as Python package directories. In Python 3.3 and later, this file is not strictly required for a directory to be considered a package.
29. Explain the use of the collections
module in Python.
The collections
module provides specialized container datatypes such as Counter
, deque
, OrderedDict
, defaultdict
, and namedtuple
.
30. How does list slicing work in Python?
List slicing allows you to access a subset of the list. The syntax is list[start:stop:step]
. Example:
numbers = [1, 2, 3, 4, 5]
print(numbers[1:4]) # Output: [2, 3, 4]
31. What are metaclasses in Python?
Metaclasses are classes of classes. They define how classes behave. A class is an instance of a metaclass.
32. How can you handle multiple exceptions in a single except
block?
You can handle multiple exceptions in a single except
block by specifying a tuple of exceptions. Example:
try:
# code that may raise exceptions
except (TypeError, ValueError) as e:
# handle exceptions
33. What are the different methods to copy an object in Python?
In Python, you can copy an object using:
- Shallow Copy: Using the
copy()
method from thecopy
module.
import copy
shallow_copy = copy.copy(original)
- Deep Copy: Using the
deepcopy()
method from thecopy
module.
import copy
deep_copy = copy.deepcopy(original)
34. What are the key differences between lists and tuples in Python?
- Mutability: Lists are mutable (they can be changed), while tuples are immutable (they cannot be changed once created).
- Syntax: Lists are created using square brackets
[]
, while tuples are created using parentheses()
. - Performance: Tuples can be faster than lists due to their immutability.
35. What is the purpose of self
in Python classes?
self
is a reference to the current instance of the class and is used to access variables and methods associated with that instance. It must be the first parameter of any method in the class.
36. Explain how you can implement a singleton pattern in Python.
A singleton pattern ensures a class has only one instance and provides a global point of access to it. This can be implemented using:
- A class with a method returning the singleton instance:
class Singleton:
_instance = None
def __new__(cls, *args, **kwargs):
if not cls._instance:
cls._instance = super(Singleton, cls).__new__(cls, *args, **kwargs)
return cls._instance
37. What is the difference between staticmethod
and classmethod
in Python?
- staticmethod: A method that does not receive an implicit first argument. It is bound to the class and not the instance.
class MyClass:
@staticmethod
def static_method():
print("Static method called")
- classmethod: A method that receives the class as the first argument. It can modify class state that applies across all instances of the class.
class MyClass:
@classmethod
def class_method(cls):
print("Class method called")
38. What is a metaclass in Python and how do you use it?
A metaclass is a class of a class that defines how a class behaves. Metaclasses are used to create classes in Python and can be specified using the metaclass
keyword.
class MyMeta(type):
def __new__(cls, name, bases, dct):
print("Creating class:", name)
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
39. Explain the difference between @staticmethod
and @classmethod
.
- @staticmethod: A decorator to define a static method. It does not receive an implicit first argument.
- @classmethod: A decorator to define a class method. It receives the class as the first argument.
40. What are Python’s built-in data structures?
Python’s built-in data structures include:
- List: Ordered and mutable collection of items.
- Tuple: Ordered and immutable collection of items.
- Set: Unordered collection of unique items.
- Dictionary: Unordered collection of key-value pairs.
41. How do you implement error handling in Python?
Error handling in Python is implemented using try
, except
, else
, and finally
blocks. Example:
try:
# Code that might raise an exception
except SomeException as e:
# Code to handle the exception
else:
# Code to execute if no exception occurs
finally:
# Code to execute regardless of whether an exception occurs or not
42. What is the difference between the __str__
and __repr__
methods in Python?
__str__
: Returns a string representation of the object, meant to be readable.__repr__
: Returns a string that would ideally be a valid Python expression to recreate the object, meant to be unambiguous.
43. What is the use of the yield
keyword in Python?
The yield
keyword is used to create a generator. Generators allow you to iterate through a set of values without creating and storing the entire list in memory.
def my_generator():
yield 1
yield 2
yield 3
44. What is the __init__.py
file used for in Python?
The __init__.py
file is used to mark directories on disk as Python package directories. It can also be used to initialize package-level variables.
45. Explain the concept of duck typing in Python.
Duck typing is a concept related to dynamic typing in Python where the type or class of an object is less important than the methods it defines. If an object “quacks like a duck and walks like a duck,” it can be used in place of a duck.
class Duck:
def quack(self):
print("Quack")
class Person:
def quack(self):
print("I'm quacking like a duck")
def in_the_forest(duck):
duck.quack()
duck = Duck()
person = Person()
in_the_forest(duck) # Quack
in_the_forest(person) # I'm quacking like a duck
46. How do you work with dates and times in Python?
Python provides the datetime
module to work with dates and times. Example:
import datetime
now = datetime.datetime.now()
print(now.strftime("%Y-%m-%d %H:%M:%S"))
47. What are comprehensions in Python?
Comprehensions provide a concise way to create lists, sets, or dictionaries. Examples:
- List comprehension:
squares = [x**2 for x in range(10)]
- Set comprehension:
unique_squares = {x**2 for x in range(10)}
- Dictionary comprehension:
square_dict = {x: x**2 for x in range(10)}
48. Explain the purpose of the __call__
method in Python.
The __call__
method allows an instance of a class to be called as a function. Example:
class MyCallable:
def __call__(self, *args, **kwargs):
print("Instance called with", args, kwargs)
my_callable = MyCallable()
my_callable(1, 2, 3, key="value")
49. How can you read and write files in Python?
You can read and write files using the open()
function with different modes such as 'r'
for reading and 'w'
for writing.
# Reading a file
with open('file.txt', 'r') as file:
content = file.read()
# Writing to a file
with open('file.txt', 'w') as file:
file.write("Hello, World!")
50. How do you manage dependencies and environments in Python projects?
Dependencies and environments in Python projects are managed using tools like pip
for installing packages and virtualenv
or conda
for creating isolated environments. Using pipenv
can also help manage dependencies and virtual environments together.
Conclusion
Preparing for a Python interview requires a thorough understanding of both basic and advanced concepts. Whether you are a fresher or an experienced professional, understanding these key Python interview questions and answers pdf will help you demonstrate your proficiency and confidence during the interview process. Good luck with your preparation!
If you looking for Java 8 interview preparation materials then click here
You can down Python Interview Questions and Answers pdf here